vs qt中使用checkbox选中之后在qlabel上的图像中出现方框用来截取图片,方框可用鼠标编辑大小,展示代码
时间: 2024-11-25 15:14:02 浏览: 5
在Qt中,你可以使用QGraphicsView和QGraphicsScene以及相关的图形控件来实现在QLabel上显示图片并创建可拖动的矩形选择区域。这里是一个简单的示例,展示了如何做到这一点:
```cpp
#include <QApplication>
#include <QWidget>
#include <QLabel>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QGraphicsRectItem>
#include <QPainter>
class MyWidget : public QWidget {
public:
MyWidget(QWidget* parent = nullptr) : QWidget(parent) {
QLabel* imageLabel = new QLabel(this);
// 设置初始图片
QPixmap pixmap("your_image_path.jpg");
imageLabel->setPixmap(pixmap);
QGraphicsView* graphicsView = new QGraphicsView(imageLabel, this);
graphicsView->setScene(&scene);
scene.setSceneRect(QRectF(0, 0, pixmap.width(), pixmap.height()));
QGraphicsRectItem* selectionRect = new QGraphicsRectItem(QRectF(0, 0, 0, 0), &scene);
selectionRect->setPen(Qt::red); // 红色边框
selectionRect->setFlags(QGraphicsItem::ItemIsMovable | QGraphicsItem::ItemSendsGeometryChanges);
connect(selectionRect, &QGraphicsRectItem::scenePosChanged,
this, &MyWidget::updateSelectionRectPosition);
updateSelectionRectPosition(selectionRect->scenePos());
}
private slots:
void updateSelectionRectPosition(const QPointF& pos) {
QRectF rect(pos.x(), pos.y(), selectionRect->width(), selectionRect->height());
painter().drawRect(rect);
QLabel* label = qobject_cast<QLabel*>(sender()->parent()); // 获取QLabel,以便获取其位图
if (label) {
QImage croppedImage(label->pixmap().rect(rect).toImage());
// 在这里处理或更新croppedImage,并设置到imageLabel
// label->setPixmap(QPixmap::fromImage(croppedImage));
}
}
private:
QGraphicsScene scene;
QLabel* imageLabel;
QGraphicsRectItem* selectionRect;
QPainter painter; // 用于画出矩形选择区
};
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
MyWidget widget;
widget.show();
return app.exec();
}
```
在这个例子中,我们创建了一个`QGraphicsRectItem`来代表选择区域,并连接了它的位置变化信号到`updateSelectionRectPosition`槽函数,该函数会在矩形移动时更新并绘制选择区域。然后通过`QPainter`在`QLabel`上画出选择的矩形。
请注意,实际的`croppedImage`处理和设置需要你自己实现,这里只是一个基础框架。另外,确保你的程序包含了适当的路径到图片文件"your_image_path.jpg"。
阅读全文
相关推荐
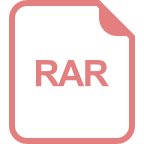
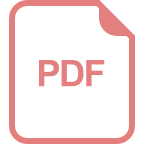
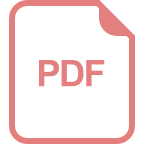

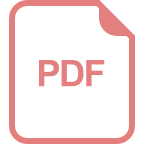
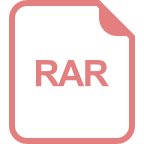
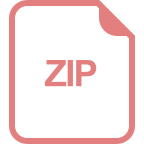
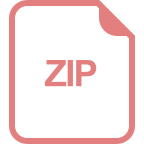
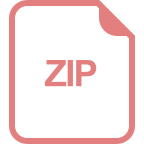
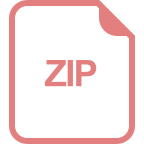
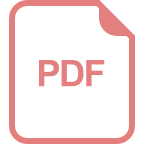
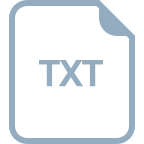
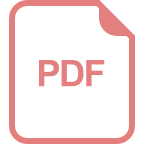
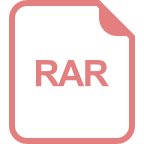
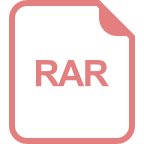
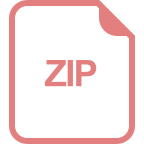