使用Java,定义一个方程类,求方程 的两个实数根,请将测试主类和方程类定义在不同的包中
时间: 2024-10-12 08:12:33 浏览: 19
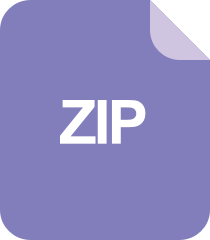
基于Java关于二元一次方程求根源码.zip
在Java中,你可以创建一个名为`Equation`的类来表示一般的二次方程,例如ax^2 + bx + c = 0,并提供方法来计算它的两个实数根。首先,我们将这个类定义在一个包`com.example.math.equations`中:
```java
// com/example/math/equations/Equation.java
package com.example.math.equations;
public class Equation {
private double a;
private double b;
private double c;
public Equation(double a, double b, double c) {
this.a = a;
this.b = b;
this.c = c;
}
// 计算判别式
private double discriminant() {
return Math.pow(b, 2) - 4 * a * c;
}
// 求解根公式,考虑正负判别式的情况
public double[] solveRoots() {
double discriminantSign = discriminant();
if (discriminantSign > 0) {
return new double[]{(-b + Math.sqrt(discriminant())) / (2 * a), (-b - Math.sqrt(discriminant())) / (2 * a)};
} else if (discriminantSign == 0) {
return new double[]{-b / (2 * a)};
} else { // 一元一次方程(复数根)
double realPart = -b / (2 * a);
double imaginaryPart = Math.sqrt(-discriminantSign) / (2 * a);
return new double[]{realPart, realPart + imaginaryPart * 1j, realPart - imaginaryPart * 1j}; // 返回复数形式
}
}
}
```
接下来,我们创建一个测试主类`Main`,位于另一个包`com.example.math.test`中,用于测试`Equation`类:
```java
// com/example/math/test/Main.java
package com.example.math.test;
import com.example.math.equations.Equation;
public class Main {
public static void main(String[] args) {
Equation equation = new Equation(1, -3, 2); // 例如求解x^2 - 3x + 2 = 0
double[] roots = equation.solveRoots();
System.out.println("First root: " + roots[0]);
System.out.println("Second root: " + roots[1]);
// 测试其他情况
equation = new Equation(1, 0, 1);
roots = equation.solveRoots();
System.out.println("For x^2 + 1 = 0, the result is: " + roots[0]); // 只有一个实数根
}
}
```
在这个例子中,`Main`类不是专门用来测试`Equation`类的,但它展示了如何使用`Equation`类。为了运行这个程序,你需要设置合适的包结构并在IDE中分别构建这两个包。
阅读全文
相关推荐
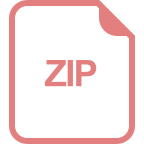
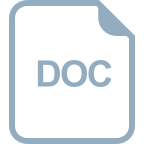
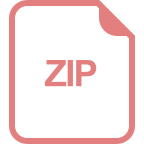
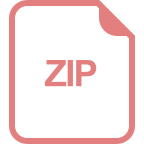
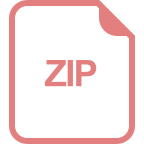
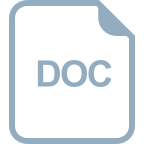
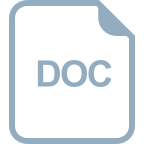
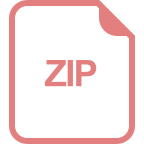
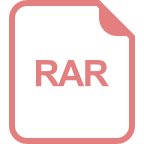
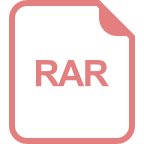
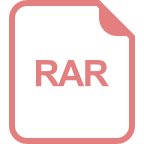
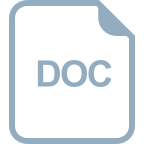
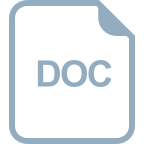