线性表的顺序表示和实现C语言代码
时间: 2024-09-24 07:09:53 浏览: 58
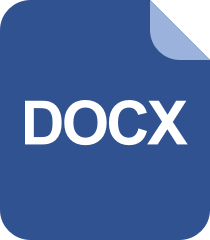
线性表顺序实现与操作详解-C语言实战应用
线性表是一种基本的数据结构,它是一系列元素的集合,其中每个元素都有一个唯一的索引或位置。顺序表是指通过连续的内存空间存储数据,每个元素的位置可以通过下标直接访问。
在C语言中,我们可以使用数组来实现顺序表。以下是顺序表的基本实现:
```c
#include <stdio.h>
// 定义线性表节点结构体
typedef struct {
int data; // 数据域
int index; // 下标,用于内部查找速度较慢
} Node;
// 顺序表类型定义
typedef struct {
Node* list; // 指向首节点的指针
int size; // 当前表大小
int capacity; // 表的最大容量
} LinearList;
// 初始化顺序表
void init_list(LinearList* lst) {
lst->list = NULL;
lst->size = 0;
lst->capacity = 0;
}
// 插入元素到列表尾部
void insert_at_end(LinearList* lst, int value) {
if (lst->size == lst->capacity) {
resize_list(lst);
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->index = lst->size;
newNode->next = NULL;
lst->list[lst->size++] = *newNode;
}
// 删除指定位置的元素
void delete_at_index(LinearList* lst, int index) {
if (index >= 0 && index < lst->size) {
Node* temp = lst->list[index];
for (int i = index; i < lst->size - 1; i++) {
lst->list[i] = lst->list[i + 1];
}
lst->list[lst->size - 1] = NULL;
free(temp);
lst->size--;
} else {
printf("Index out of range.\n");
}
}
// 打印顺序表
void print_list(LinearList* lst) {
Node* current = lst->list;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 动态扩容函数
void resize_list(LinearList* lst) {
lst->capacity *= 2;
Node* newList = (Node*)realloc(lst->list, lst->capacity * sizeof(Node));
lst->list = newList;
}
// 示例
int main() {
LinearList myList;
init_list(&myList);
insert_at_end(&myList, 10);
insert_at_end(&myList, 20);
print_list(&myList); // 输出: 10 20
return 0;
}
```
阅读全文
相关推荐
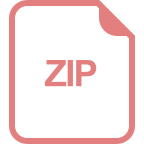
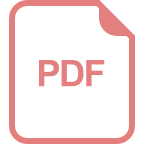
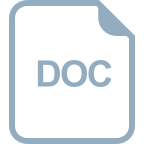
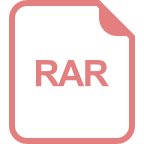
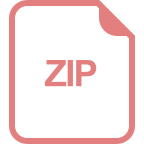
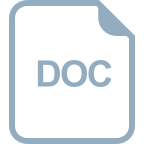



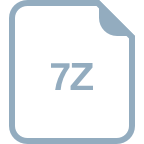
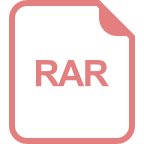
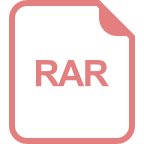
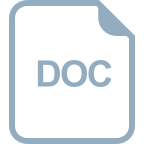
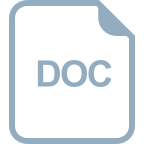
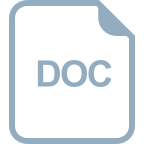
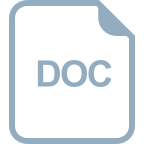
