提供这些问题的python代码
时间: 2024-09-19 12:03:58 浏览: 34
当然,这里我会提供一些基本的问题和对应的Python代码示例:
**1. 线性回归模型的创建及拟合**
```python
from sklearn.linear_model import LinearRegression
# 假设 X_train 和 y_train 是训练数据,shape应该是(n_samples, n_features)
model = LinearRegression() # 创建模型实例
model.fit(X_train, y_train) # 使用训练数据拟合模型
# 预测新数据点 price
new_data = [[some_new_features]]
predicted_price = model.predict(new_data)
```
**2. 计算并画出梯度下降的学习曲线**
```python
import numpy as np
import matplotlib.pyplot as plt
# 假设 loss_history 是每次迭代后的损失值列表
plt.plot(loss_history, label='Loss')
plt.xlabel('Iterations')
plt.ylabel('Loss')
plt.title('Gradient Descent Learning Curve')
plt.legend()
plt.show()
```
**3. 梯度下降算法的伪代码实现**
```python
def gradient_descent(X, y, theta, alpha, num_iterations):
m = len(y)
for i in range(num_iterations):
hypothesis = np.dot(X, theta)
error = hypothesis - y
gradient = (1/m) * np.dot(X.T, error)
theta = theta - alpha * gradient
return theta
```
请注意,以上代码片段仅用于演示基本概念,并未涵盖所有可能的异常处理和优化。在实际应用中,可能还需要添加更多的细节和错误检查。
阅读全文
相关推荐
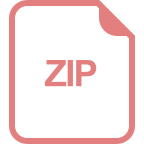
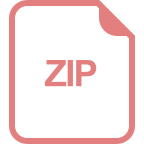
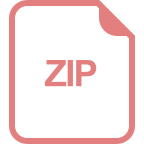
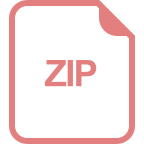
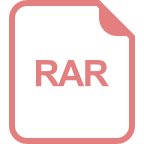
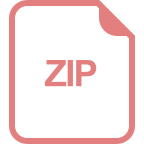