qt如何用系统时间来做一个计时的标志,信号灯的值在收到变化量后过两秒再变成当前值对应的信号颜色,不足两秒就保持原来的值对应的信号灯不变,仅在显示的时候有这一操作
时间: 2024-09-25 10:19:59 浏览: 27
在Qt中,你可以使用`QTimer`配合`QObject`的槽函数和信号机制来实现这个功能。首先,你需要创建一个`QObject`的子类,比如`SignalLight`,在这个类中定义一个表示时间差的变量、一个定时器以及状态相关的成员变量。
```cpp
class SignalLight : public QObject {
public:
explicit SignalLight(QObject *parent = nullptr)
: QObject(parent), timer_(this) {
connect(&timer_, &QTimer::timeout, this, &SignalLight::updateColor);
}
private slots:
void updateColor() {
qint64 currentTime = QDateTime::currentMSecsSinceEpoch();
qint64 twoSecondsAgo = currentTime - 2000; // 2000毫秒等于2秒
if (currentValue < twoSecondsAgo) {
// 如果时间差小于2秒,维持原色
emit colorChanged(currentColor());
} else {
// 时间差大于或等于2秒,更新到当前时间对应的信号颜色
emit colorChanged(QColor::fromRgb(...)); // 代入实际的颜色值
resetTimer(); // 重置定时器
}
}
private:
QTimer timer_;
qint64 currentValue;
// 添加其他信号和槽函数,如colorChanged(const QColor& color) 和 resetTimer()
// 初始化和设置信号颜色的方法
void setColorAndStartTimer(QColor color) {
currentValue = QDateTime::currentMSecsSinceEpoch();
setcurrentColor(color);
timer_.start(1000); // 定义每秒检查一次的时间间隔
}
// 清除定时器并停止计时
void resetTimer() {
timer_.stop();
currentValue = 0;
}
};
```
然后,在需要计时的场景下,你可以创建`SignalLight`实例,并调用`setColorAndStartTimer()`方法:
```cpp
SignalLight signalLight;
// 设置初始颜色
QColor initialColor = ...;
signalLight.setColorAndStartTimer(initialColor);
// 当接收到时间变化的信号(这里假设有个名为timeUpdated的信号)
void handleTimeUpdate(qint64 newTime) {
qint64 timeDiff = newTime - signalLight.currentValue;
if (timeDiff > 2000) {
// 如果时间差超过2秒,改变颜色并启动新计时
QColor newColor = ...; // 新的颜色
signalLight.setColorAndStartTimer(newColor);
} else {
// 否则,只更新颜色值,无需启动新的计时
signalLight.setcurrentColor(newColor);
}
}
```
阅读全文
相关推荐
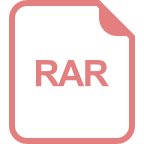
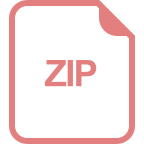
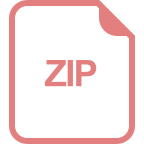




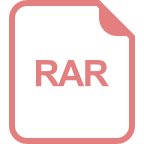
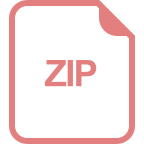
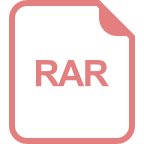
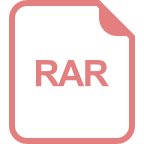
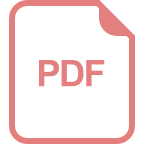
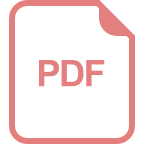