c语言中的round
时间: 2024-12-24 14:23:08 浏览: 7
在C语言中,没有内置的`round`函数用于四舍五入到最接近的整数。但是,你可以通过组合数学运算和条件判断来实现类似的功能。例如,可以使用`<`(小于)、`>`(大于)运算符以及`fmod`(求余)函数来自定义一个简单的四舍五入规则。
这里是一个简单的示例,如果需要将浮点数`x`向上圆(即对正数保留小数点后第一位,对负数取整),可以这样做:
```c
#include <math.h>
double round_up(double x) {
if (x >= 0)
return floor(x + 0.5);
else
return ceil(x); // 对负数取整
}
// 或者如果你只需要整数结果,可以去掉 `+0.5`
int int_round_up(double x) {
return (x > 0) ? (int)(x + 0.5) : (int)x;
}
```
如果你想得到向下或精确到特定位数的四舍五入,你需要使用其他方法,比如`lrint`(Linux系统)或自己编写更复杂的逻辑。
请注意,在C标准库中,对于标准的四舍五入功能,你需要引入`<math.h>`头文件并使用`rint()`或`lrint()`函数。
相关问题
c语言中round函数用法
round函数是C语言中的一个数学函数,用于对浮点数进行四舍五入运算。它的原型定义在math.h头文件中,函数的返回值类型为double类型。round函数的用法如下:
double round(double x);
其中,x为需要进行四舍五入运算的浮点数。函数返回最接近x的整数值,如果x与两个整数的距离相等,则返回偶数整数。如果出现错误,函数返回0.0。
例如,round(2.499999)的结果为2,round(-2.499999)的结果为-2,roundf(2.8f)的结果为3,roundf(-2.8f)的结果为-3,roundl(2.5)的结果为2,roundl(-2.5)的结果为-2。
c语言中round函数的用法
在 C 语言中,`round()` 函数是一个数学库函数,它用于对给定的浮点数进行四舍五入。这个函数的原型通常定义在 `<math.h>` 头文件里,其基本语法如下:
```c
double round(double x);
```
这里的 `x` 是需要进行四舍五入的数值,`round(x)` 返回的是最接近 `x` 的整数。如果 `x` 离两个整数等距,那么规则是向正无穷大方向(即偶数部分大于等于0.5则向上取整,小于0.5则向下取整)进行四舍五入。
例如:
```c
#include <stdio.h>
#include <math.h>
int main() {
double num = 3.7;
int rounded_num = round(num);
printf("Rounded %f to nearest integer is %d\n", num, rounded_num);
num = -3.6;
rounded_num = round(num);
printf("Rounded %f to nearest integer is %d\n", num, rounded_num);
return 0;
}
```
运行上述程序,你会看到:
```
Rounded 3.700000 to nearest integer is 4
Rounded -3.600000 to nearest integer is -4
```
阅读全文
相关推荐
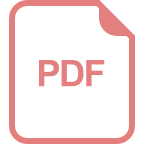
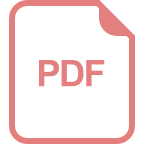
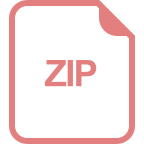













