C# FileStream 共享文件
时间: 2023-11-23 18:51:24 浏览: 110
C#的FileStream类可以用于读写文件,同时它也支持共享文件的操作。使用FileStream类操作共享文件需要指定FileShare枚举类型中的参数。
举个例子,如果你想以只读的方式打开一个共享文件,可以使用以下代码:
```
FileStream fs = new FileStream("文件路径", FileMode.Open, FileAccess.Read, FileShare.Read);
```
在这个例子中,FileShare.Read指定了共享方式为只读。
相关问题
C# 写入共享文件
在C#中,你可以使用FileStream类打开一个共享访问的流,以便其他进程也可以访问该文件。你可以指定FileShare枚举以允许其他进程以只读或读写模式访问该文件。
以下是一个写入共享文件的示例代码:
```csharp
using System;
using System.IO;
using System.Text;
class Program
{
static void Main(string[] args)
{
string filePath = "C:\\temp\\myfile.txt";
string data = "Hello, World!";
byte[] bytes = Encoding.UTF8.GetBytes(data);
try
{
using (FileStream fileStream = new FileStream(filePath, FileMode.Append, FileAccess.Write, FileShare.ReadWrite))
{
fileStream.Write(bytes, 0, bytes.Length);
}
}
catch (Exception e)
{
Console.WriteLine("An error occurred: " + e.Message);
}
}
}
```
在上面的示例代码中,我们使用了FileStream类打开了一个名为"myfile.txt"的文件,并指定了FileShare.ReadWrite枚举以允许其他进程以只读或读写模式访问该文件。然后,我们使用Write方法将数据写入到文件中。
需要注意的是,如果其他进程正在读取或写入该文件,你可能无法立即访问该文件。此外,如果你尝试在打开文件的过程中删除该文件,将会抛出一个IOException异常。因此,你应该始终在写入文件之前检查文件是否存在,并且在写入完成后关闭文件流。
c# 传递用户名密码访问共享文件服务器上的文件
在C#中,为了通过用户名和密码访问共享文件服务器上的文件,通常会涉及到网络文件系统(Network File System,NFS)或Windows网络共享功能。你可以使用`System.IO.Packaging`库来操作网络文件,或者直接通过`System.Net.WebClient`或`System.Net.Sockets`进行低级操作。这里是一个基本的步骤说明:
1. **使用WebClient**:
```csharp
using System.Net;
using System.IO;
string userName = "your_username";
string password = "your_password";
string serverPath = "\\server_address\\shared_folder";
var client = new WebClient();
client.Credentials = new NetworkCredential(userName, password);
try {
byte[] fileBytes = client.DownloadData(serverPath);
// 将文件字节数组保存到本地或其他位置
File.WriteAllBytes("local_file_path", fileBytes);
} catch (Exception ex) {
Console.WriteLine($"Error accessing file: {ex.Message}");
}
```
2. **使用DirectoryInfo和FileStream**:
```csharp
using System.IO;
using System.Security.Principal;
string username = "your_username";
string password = "your_password";
string filePath = "\\server_address\\shared_folder\\file.txt";
// 创建一个Principal对象用于身份验证
var identity = new GenericPrincipal(new GenericIdentity(username), null);
// 使用Credentials获取安全上下文
var credentials = new NetworkCredential(username, password);
var securityContext = new SafeHandleInheritable(credentials.GetCredential(NetworkAuthentication.DefaultHost, "NTLM").GetNativeObject(), true);
using (FileStream fs = File.OpenRead(filePath, FileAccess.Read, FileShare.Read))
using (Stream networkStream = new NetworkStream(fs, securityContext)) {
// 读取文件内容...
}
```
注意:这些示例假设你正在使用Windows环境,并且共享文件服务器支持NTLM或Kerberos认证。在实际项目中,还需要考虑异常处理和权限管理。
阅读全文
相关推荐
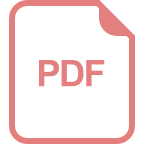
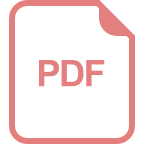
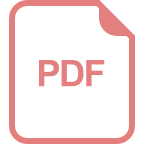
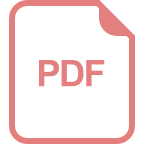
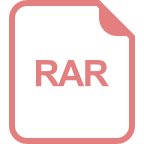
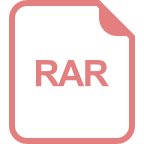
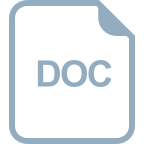
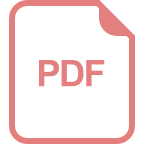
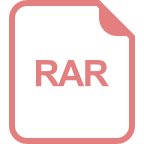
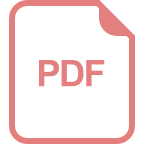
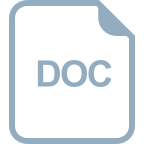


