c语言实现两个升序链表合并为一个降序链表
时间: 2023-07-17 10:12:22 浏览: 69
下面是用 C 语言实现将两个升序链表合并为一个降序链表的示例代码:
```c
#include<stdio.h>
#include<stdlib.h>
struct ListNode {
int val;
struct ListNode* next;
};
struct ListNode* mergeLists(struct ListNode* l1, struct ListNode* l2) {
if (l1 == NULL) {
return l2;
}
if (l2 == NULL) {
return l1;
}
struct ListNode* merged_head;
if (l1->val < l2->val) {
merged_head = l1;
merged_head->next = mergeLists(l1->next, l2);
} else {
merged_head = l2;
merged_head->next = mergeLists(l1, l2->next);
}
return merged_head;
}
void printList(struct ListNode* head) {
struct ListNode* current = head;
while (current != NULL) {
printf("%d ", current->val);
current = current->next;
}
printf("\n");
}
int main() {
// 创建链表1:1 -> 3 -> 5
struct ListNode* l1 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* l1_1 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* l1_2 = (struct ListNode*)malloc(sizeof(struct ListNode));
l1->val = 1;
l1_1->val = 3;
l1_2->val = 5;
l1->next = l1_1;
l1_1->next = l1_2;
l1_2->next = NULL;
// 创建链表2:2 -> 4 -> 6
struct ListNode* l2 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* l2_1 = (struct ListNode*)malloc(sizeof(struct ListNode));
struct ListNode* l2_2 = (struct ListNode*)malloc(sizeof(struct ListNode));
l2->val = 2;
l2_1->val = 4;
l2_2->val = 6;
l2->next = l2_1;
l2_1->next = l2_2;
l2_2->next = NULL;
// 合并链表并打印结果
struct ListNode* merged = mergeLists(l1, l2);
printList(merged);
// 释放内存
free(l1);
free(l1_1);
free(l1_2);
free(l2);
free(l2_1);
free(l2_2);
return 0;
}
```
在这个示例中,我们首先定义了一个 `ListNode` 结构体来表示链表的节点。然后,实现了 `mergeLists` 函数来完成链表的合并。最后,在 `main` 函数中创建了两个升序链表,调用 `mergeLists` 函数进行合并,并打印结果。
请注意,在实际使用时,应该根据需要自行定义释放链表内存的函数,以避免内存泄漏。这里只是简单示例,没有包含完整的内存释放操作。
相关推荐
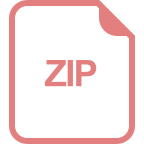
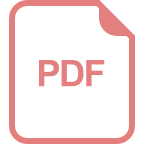














