java 代码获取zip压缩后的字符数组,如何获取其中内容
时间: 2024-12-02 18:17:30 浏览: 1
在Java中,你可以使用java.util.zip库提供的功能来处理Zip文件。如果你想将ZIP文件转换为字符数组,首先你需要读取文件的内容,然后将字节数据转换为字符。这里是一个简单的步骤:
```java
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class ZipToCharArray {
public static char[] getZipContentAsChars(String zipFilePath, String entryName) throws IOException {
byte[] bytes = readZipFile(zipFilePath);
ByteArrayOutputStream byteArrayOut = new ByteArrayOutputStream();
unzipEntry(bytes, entryName, byteArrayOut);
return byteArrayOut.toString("UTF-8").toCharArray();
}
private static byte[] readZipFile(String filePath) throws IOException {
byte[] bytes = Files.readAllBytes(Paths.get(filePath));
return bytes;
}
private static void unzipEntry(byte[] zipData, String entryName, ByteArrayOutputStream outputStream) throws IOException {
try (ZipInputStream zis = new ZipInputStream(new ByteArrayInputStream(zipData))) {
ZipEntry ze = zis.getNextEntry();
while (ze != null) {
if (ze.getName().equals(entryName)) {
byte[] buffer = new byte[1024];
int length;
while ((length = zis.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
break; // Assuming you only want the content of one entry
}
ze = zis.getNextEntry();
}
}
}
}
```
在这个例子中,`getZipContentAsChars`方法接受ZIP文件路径和需要提取的entry名称,读取整个ZIP文件,找到指定的entry,并将其解压到ByteArrayOutputStream中,最后转换成字符数组。
阅读全文
相关推荐
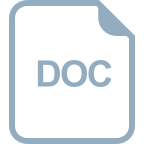
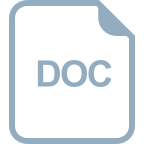
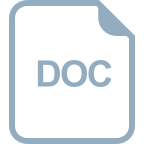
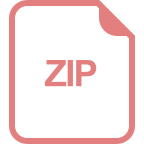
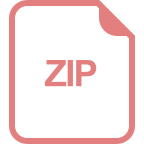
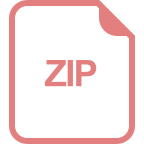
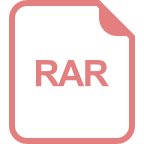
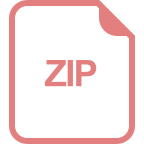
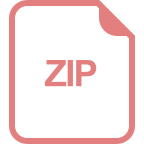
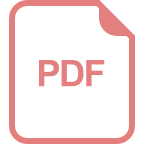
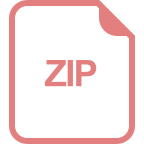
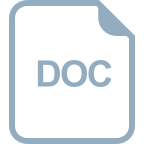
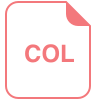
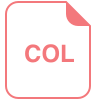
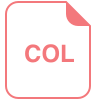
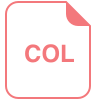
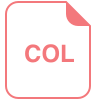
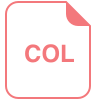
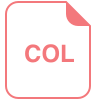