cpp opencv 以灰度图形式读图
时间: 2024-09-10 08:23:59 浏览: 53
在C++中使用OpenCV读取图像并转换为灰度图,通常涉及以下步骤:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
// 读取彩色图像
cv::Mat loadColorImage(const char* filename) {
cv::Mat img = cv::imread(filename);
if (img.empty()) {
std::cerr << "Could not open or find the image." << std::endl;
exit(-1);
}
return img;
}
// 转换为灰度图像
cv::Mat toGrayscale(cv::Mat& color_img) {
cv::Mat gray_img;
cv::cvtColor(color_img, gray_img, cv::COLOR_BGR2GRAY);
return gray_img;
}
int main() {
// 读取彩色图片文件
const char* img_path = "path_to_your_image.jpg";
cv::Mat color_img = loadColorImage(img_path);
// 转换为灰度
cv::Mat gray_img = toGrayscale(color_img);
// 检查灰度图像是否成功创建
std::cout << "Gray image size: " << gray_img.size() << std::endl;
// 灰度图像可用于后续处理
// ...
return 0;
}
```
在这个代码片段中,首先通过`cv::imread`函数加载彩色图像,如果图像无法打开则返回空矩阵。接着,`cv::cvtColor`函数用于将BGR色彩空间的图像转换为灰度,生成一个新的灰度图像。
阅读全文
相关推荐
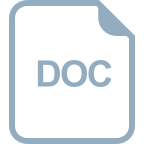
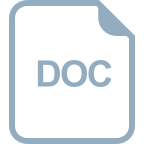
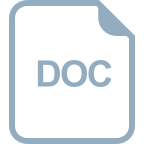

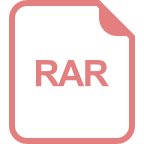
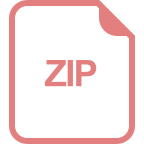
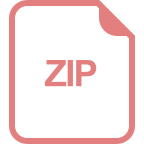
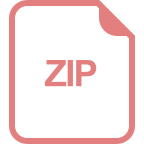
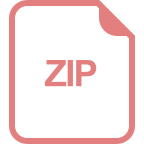
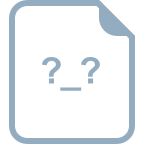
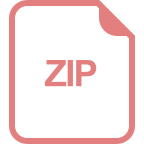
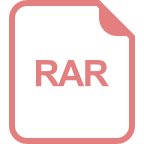
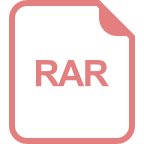
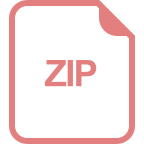
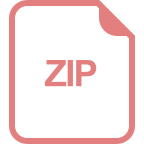
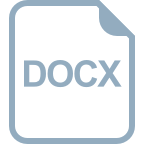
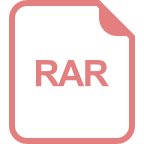