输入三个数,找出最大值,用c++写
时间: 2024-10-25 22:08:41 浏览: 16
在C++中,你可以通过比较的方式来找到输入的三个数中的最大值。这里是一个简单的示例程序:
```cpp
#include <iostream>
int findMax(int a, int b, int c) {
if (a > b && a > c) {
return a;
} else if (b > a && b > c) {
return b;
} else {
return c;
}
}
int main() {
int num1, num2, num3;
std::cout << "请输入第一个数: ";
std::cin >> num1;
std::cout << "请输入第二个数: ";
std::cin >> num2;
std::cout << "请输入第三个数: ";
std::cin >> num3;
int maxNum = findMax(num1, num2, num3);
std::cout << "这三个数的最大值是: " << maxNum << std::endl;
return 0;
}
```
在这个程序里,`findMax`函数接受三个整数作为参数,通过一系列if...else条件判断来确定并返回最大的那个数。在`main`函数中,用户会被提示输入三个数字,然后结果会被打印出来。
相关问题
c++从用户输入中读取三个数字并找出三个数字最大值
在C++中,你可以通过使用`cin`(标准输入流)从用户处获取三个数字,并使用条件比较来找到其中的最大值。下面是一个简单的示例程序:
```cpp
#include <iostream>
using namespace std;
int main() {
int num1, num2, num3;
cout << "请输入第一个数字: ";
cin >> num1;
cout << "请输入第二个数字: ";
cin >> num2;
cout << "请输入第三个数字: ";
cin >> num3;
// 比较并找出最大值
int max_value = (num1 > num2) ? ((num1 > num3) ? num1 : num3) : ((num2 > num3) ? num2 : num3);
cout << "这三个数字中的最大值是: " << max_value << endl;
return 0;
}
```
在这个程序中,我们首先声明了三个整数变量`num1`, `num2`, 和 `num3`,然后依次从用户那里读取输入。接着使用三元运算符(条件表达式)来比较它们之间的大小,最后将结果存储在`max_value`变量中并输出。
c++从用户输入中读取三个数字并找出三个数字最大值限时三秒
在C++中,如果你想让用户输入三个数字并在限定时间内找到它们的最大值,你可以使用`chrono`库来实现计时功能。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <chrono> // 引入时间戳库
using namespace std;
int main() {
int num1, num2, num3;
cout << "请输入三个数字(三秒倒计时): ";
auto start = chrono::high_resolution_clock::now(); // 获取开始时间
cin >> num1 >> num2 >> num3; // 从用户输入读取
auto end = chrono::high_resolution_clock::now(); // 获取结束时间
auto duration = chrono::duration_cast<chrono::seconds>(end - start); // 计算时间差
if (duration.count() <= 3) { // 如果时间少于或等于3秒
int max_num;
if (num1 > num2 && num1 > num3) {
max_num = num1;
} else if (num2 > num1 && num2 > num3) {
max_num = num2;
} else {
max_num = num3;
}
cout << "三个数字的最大值是: " << max_num << endl;
} else {
cout << "输入超时了!" << endl;
}
return 0;
}
```
在这个例子中,我们首先获取用户的输入,然后计算从开始到结束的时间差。如果时间差小于或等于3秒,我们就找出并显示这三个数字中的最大值;否则,提示用户输入超时。
阅读全文
相关推荐
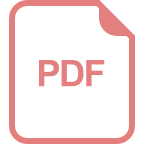
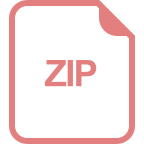
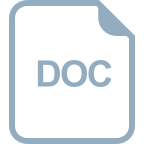
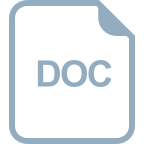








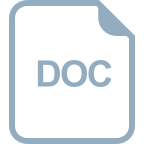
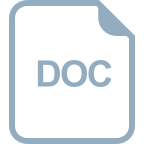


