vue3 头部导航栏点击调用对应路由组件的方法打开弹窗
时间: 2024-09-25 22:19:43 浏览: 56
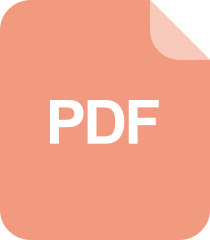
Vue结合路由配置递归实现菜单栏功能
在Vue3中,为了实现头部导航栏点击调用对应路由组件并显示弹窗的功能,通常会结合Vuex管理状态、路由器进行操作,并可能使用Element Plus库中的`el-dialog`组件来创建弹窗。以下是基本步骤:
1. **安装依赖**:首先需要安装`vue-router`和`element-plus`等必要的库。
```bash
npm install vue-router element-plus
```
2. **路由配置**:在`router/index.js`文件中,为每个路由页面添加对应的元信息,比如`meta`属性,用来存储是否显示弹窗的标志。
```js
const routes = [
{
path: '/route1',
component: () => import('@/views/Route1.vue'),
meta: { showPopup: true },
},
// 其他路由...
];
```
3. **创建Vuex store**:如果需要在多个组件间共享状态,可以使用Vuex来管理弹窗的状态。在`store/modules/popup.js`或类似模块中定义弹窗状态。
4. **导航守卫**:在`router.js`中设置全局的导航守卫,在`beforeEach`钩子中检查当前路由的`meta.showPopup`值。
```js
import { useRouter } from 'vue-router';
// ...
router.beforeEach((to, from, next) => {
const router = useRouter();
if (to.meta.showPopup) {
router.app.$emit('showPopup', to.name); // 使用自定义事件通知组件显示弹窗
}
next();
});
```
5. **组件监听事件**:在你的头部导航栏组件中监听这个自定义事件。当接收到`showPopup`事件时,你可以打开相应的弹窗组件。
```html
<template>
<header ref="header">
<!-- ... -->
<button @click="$emit('showPopup', 'dialogName')">点击显示弹窗</button>
</header>
<el-dialog v-model="isShowing" :name="dialogName" title="标题">
<div>弹窗内容...</div>
</el-dialog>
</template>
<script setup>
import { ref } from 'vue';
import { useStore } from '@/store';
import { ElDialog } from 'element-plus';
const isShowing = ref(false);
const dialogName = 'dialog1'; // 根据需要替换
function setShowPopup(name) {
isShowing.value = true;
dialogName = name;
}
useEffect(() => {
const store = useStore();
store.subscribe((mutation) => {
if (mutation.type === 'SHOW_POPUP') {
setShowPopup(mutation.payload);
}
});
}, [setShowPopup]);
</script>
```
现在当你在头部导航栏点击按钮时,对应的路由组件弹窗就会被打开。如果你有其他特定的需求,如动态加载弹窗组件,也可以调整以上代码。
阅读全文
相关推荐
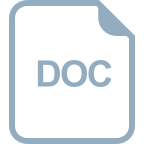
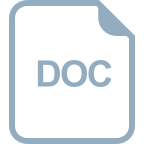
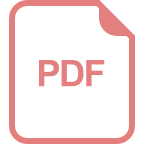
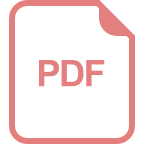
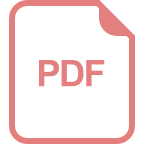
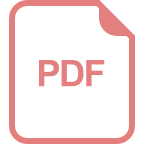
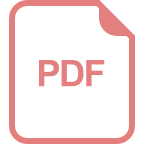
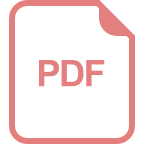
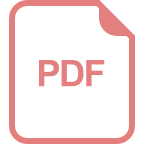
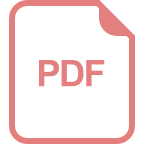
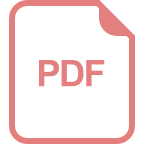
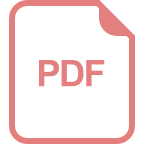
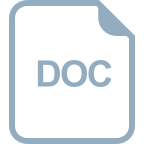




