jdbc批量insert数据
要使用JDBC进行批量插入数据,你可以按照以下步骤操作:
获取数据库连接:使用JDBC驱动程序获取与数据库的连接。例如,使用
DriverManager.getConnection()
方法。创建PreparedStatement对象:使用连接对象创建
PreparedStatement
对象,并指定插入语句,但是不指定参数值。例如,connection.prepareStatement("INSERT INTO table_name (column1, column2) VALUES (?, ?)")
。设置参数值:使用
PreparedStatement
对象的setXxx()
方法为每个参数设置值。例如,preparedStatement.setInt(1, value1)
和preparedStatement.setString(2, value2)
。添加批量操作:使用
addBatch()
方法将每个PreparedStatement
对象添加到批处理中。例如,preparedStatement.addBatch()
。执行批处理:使用
executeBatch()
方法执行批处理操作。例如,preparedStatement.executeBatch()
。关闭连接和声明对象:在操作完成后,关闭连接和所有相关的声明对象。例如,
preparedStatement.close()
和connection.close()
。
以下是一个示例代码片段,演示如何使用JDBC进行批量插入数据:
Connection connection = null;
PreparedStatement preparedStatement = null;
try {
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/database_name", "username", "password");
String insertQuery = "INSERT INTO table_name (column1, column2) VALUES (?, ?)";
preparedStatement = connection.prepareStatement(insertQuery);
// 设置参数值并添加到批处理
preparedStatement.setInt(1, value1);
preparedStatement.setString(2, value2);
preparedStatement.addBatch();
// 可以继续添加更多批处理操作
// 执行批处理
int[] batchResult = preparedStatement.executeBatch();
// 处理批处理结果
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭连接和声明对象
if (preparedStatement != null) {
try {
preparedStatement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
请注意,以上代码只是一个示例,你需要根据实际的表结构和数据来修改插入语句和参数设置。确保在使用JDBC进行数据库操作时,遵循相关的安全和最佳实践。
相关推荐

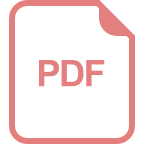














