有一个txt文件需要将其中一段内容替换为2
时间: 2024-09-26 14:07:25 浏览: 25
当你需要替换文本文件中的某段特定内容时,可以按照以下步骤操作:
1. **打开文件**: 使用适当的编程语言库(如Python的`open()`函数、Node.js的`fs`模块等),先打开你的.txt文件并读取其内容。
2. **查找和定位**: 定义你要替换的内容,并遍历文件内容,查找这一段字符串出现的位置。
3. **替换内容**: 找到目标位置后,创建一个新的字符串,将原内容替换成新的字符"2"。
4. **保存修改**: 更新文件内容至新版本,通常会关闭原有的文件流,然后再打开写入模式,写入替换后的新内容。
5. **处理异常**: 要记得捕获可能出现的错误,比如文件权限问题、文件不存在等情况。
这里是一个简单的Python示例:
```python
def replace_content_in_file(input_file, search_text, replacement):
with open(input_file, 'r') as file:
content = file.read()
# 使用正则表达式或其他方法替换
new_content = content.replace(search_text, replacement)
with open(input_file, 'w') as file:
file.write(new_content)
# 示例用法
replace_content_in_file('yourfile.txt', '旧内容', '2')
```
相关问题
文本文件a.txt中存放有一段英文文字,编写两个版本的程序,将其中的字母 a 全部替换为 A ,其它保持不变。
Python 版本:
```python
# 打开文件并读取内容
with open('a.txt', 'r') as f:
content = f.read()
# 将 a 替换为 A
new_content = content.replace('a', 'A')
# 将修改后的内容写入文件
with open('a.txt', 'w') as f:
f.write(new_content)
```
C++ 版本:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
// 打开文件并读取内容
ifstream fin("a.txt");
string content((istreambuf_iterator<char>(fin)), (istreambuf_iterator<char>()));
fin.close();
// 将 a 替换为 A
size_t pos = content.find('a');
while (pos != string::npos) {
content.replace(pos, 1, "A");
pos = content.find('a', pos + 1);
}
// 将修改后的内容写入文件
ofstream fout("a.txt");
fout << content;
fout.close();
return 0;
}
```
文本文件a.txt中存放有一段英文文字,编写两个版本的程序,将其中的字母'a'全部替换为'A',其它保持不变。
Python 版本:
```python
# 打开文件并读取内容
with open("a.txt", "r") as f:
content = f.read()
# 替换所有的'a'
new_content = content.replace("a", "A")
# 将替换后的内容写入文件
with open("a.txt", "w") as f:
f.write(new_content)
```
C++ 版本:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
// 打开文件并读取内容
ifstream infile("a.txt");
string content;
getline(infile, content, '\0');
infile.close();
// 替换所有的'a'
for (int i = 0; i < content.length(); i++) {
if (content[i] == 'a') {
content[i] = 'A';
}
}
// 将替换后的内容写入文件
ofstream outfile("a.txt");
outfile << content;
outfile.close();
return 0;
}
```
阅读全文
相关推荐
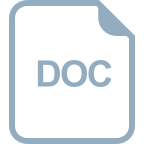
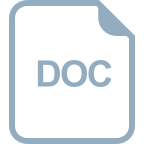
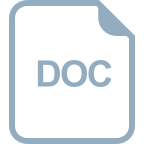













