<input class="el-input__inner" auto-complete="off" type="text" autocomplete="off" placeholder="验证码">,用Python 定位元素
时间: 2024-10-22 15:21:03 浏览: 31
在Python中,要定位HTML页面上的元素,通常可以使用Selenium库,它允许我们模拟浏览器行为并操作DOM(文档对象模型)。这里以`<input>`元素为例,假设我们想找到具有特定属性(如placeholder)的元素:
```python
from selenium import webdriver
# 创建一个新的Chrome浏览器实例
driver = webdriver.Chrome()
# 让浏览器打开网页
driver.get('https://your-url.com') # 替换为你实际的URL
# 使用CSS选择器定位带有特定placeholder的输入框
input_element = driver.find_element_by_css_selector('input[placeholder="验证码"]')
# 打印元素的一些信息
print(input_element.tag_name) # 获取标签名
print(input_element.get_attribute('value')) # 获取元素的值(如果有的话)
# 当你完成操作后,记得关闭浏览器
driver.quit()
```
请注意,这需要先安装selenium库以及对应的WebDriver(比如ChromeDriver),并且确保你的浏览器已启动。
相关问题
selenium 如何定位<div data-v-e98516b4="" class="input-btn-input el-input el-input--small"><!----><input type="text" autocomplete="off" class="el-input__inner"><!----><!----><!----><!----></div>
可以使用以下代码来定位该元素:
```
from selenium.webdriver.common.by import By
element = driver.find_element(By.CLASS_NAME, "input-btn-input")
```
或者也可以使用 CSS Selector 来定位:
```
element = driver.find_element(By.CSS_SELECTOR, "div.input-btn-input")
```
其中,`driver` 是 `webdriver` 对象。另外,这个元素的 class 属性值为 `input-btn-input`。
<input class=“el-input__inner” auto-complete=“off” type=“text” autocomplete=“off” placeholder=“验证码”>这个用什么定位写Python ui
要模拟这种HTML输入框的行为并实现类似的功能,特别是在Python UI框架(如Flask-SocketIO或Django)中,你可以通过JavaScript的AJAX请求来动态获取输入内容。在Python后端,你可以创建一个视图函数来接收这些前端发送的请求。
**在HTML模板中(假设使用Jinja2):**
```html
<form id="captcha-form">
<input class="el-input__inner"
autocomplete="off"
type="text"
id="captcha-input"
placeholder="验证码"
onclick="fetchCaptcha()">
</form>
<script>
function fetchCaptcha() {
// 使用Ajax请求,这里用的是JavaScript,实际项目中可能需要Python后端支持
$.ajax({
url: "/get_captcha", // 后端API地址
method: "GET",
success: function(response) {
document.getElementById("captcha-input").value = response.captcha;
},
error: function() {
console.error("Failed to fetch captcha");
}
});
}
</script>
```
**在Python视图(Flask示例)**:
```python
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/get_captcha')
def get_captcha():
# 这里可以生成随机验证码并返回给前端
captcha = generate_random_captcha()
return jsonify({'captcha': captcha})
# 假设generate_random_captcha是一个生成验证码的方法
# def generate_random_captcha():
# pass
if __name__ == '__main__':
app.run()
```
在这个例子中,当用户点击验证码输入框时,前端会触发`fetchCaptcha`函数,它向服务器的`/get_captcha` API发送请求以获取新的验证码。后端生成验证码并返回给前端,更新输入框的内容。
阅读全文
相关推荐
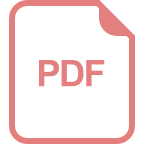
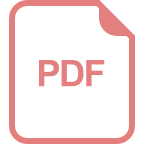
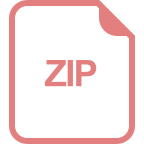









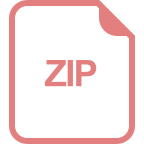
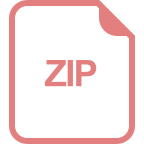
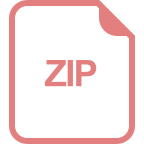
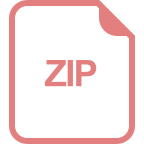