Java设计产品类、生产者类和消费者类,生成相应类的对象,生产者和消费者同时对同一产品(多个)进行生产或消费,利用线程平衡生产和消费的过程。共享缓冲区最多可以放置4个产品,生产者A和生产者B各生产多个产品;消费者1、2、3每次可以取一个产品。,输出生产者、消费者的状态。
时间: 2024-12-20 07:14:58 浏览: 12
在Java中,我们可以创建一个简单的生产者-消费者模型来模拟这个场景。首先,我们需要定义三个类:Product(产品)、Producer(生产者)和Consumer(消费者)。这里是一个简化的例子:
```java
import java.util.LinkedList;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.ReentrantLock;
// 产品类
class Product {
private static final int BUFFER_SIZE = 4;
private LinkedList<Product> buffer = new LinkedList<>();
private ReentrantLock lock = new ReentrantLock();
private Condition notFull = lock.newCondition();
private Condition notEmpty = lock.newCondition();
public synchronized void produce(Product item) throws InterruptedException {
while (buffer.size() == BUFFER_SIZE) {
notFull.await();
}
buffer.add(item);
notifyAll(); // 当生产者添加产品后,唤醒所有等待的消费者
notEmpty.signal(); // 通知消费者缓冲区有空位了
}
public synchronized Product consume() throws InterruptedException {
while (buffer.isEmpty()) {
notEmpty.await();
}
Product takenItem = buffer.removeFirst();
lock.unlock(); // 解锁缓冲区
return takenItem;
}
}
// 生产者类
class Producer implements Runnable {
private final Product product;
private int id;
public Producer(Product product, int id) {
this.product = product;
this.id = id;
}
@Override
public void run() {
for (int i = 0; i < 10; i++) { // 假设生产10个产品
try {
product.produce(new Product(id, "Product-" + id));
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Producer " + id + " produced: " + product.buffer.peek());
}
}
}
// 消费者类
class Consumer implements Runnable {
private final Product product;
private int id;
public Consumer(Product product, int id) {
this.product = product;
this.id = id;
}
@Override
public void run() {
while (true) {
try {
Product takenProduct = product.consume();
if (takenProduct != null) {
System.out.println("Consumer " + id + " consumed: " + takenProduct);
} else {
break; // 当缓冲区为空,消费者退出循环
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
System.out.println("Consumer " + id + " stopped consuming.");
}
}
```
在这个例子中,我们创建两个生产者和三个消费者。每个生产者和消费者都会作为线程运行,交替进行生产或消费操作。当缓冲区满时,生产者会等待,直到有消费者消费了一个产品;同样,当缓冲区空时,消费者会等待,直到有新的产品到来。
运行这个程序时,你会看到生产者和消费者之间的交互以及缓冲区的状态变化。这有助于理解如何通过线程和同步机制平衡生产与消费。如果你需要更详细的代码示例,我可以提供。
阅读全文
相关推荐
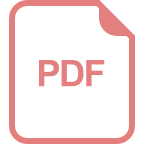
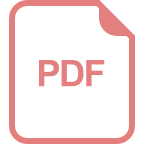
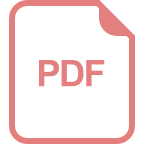


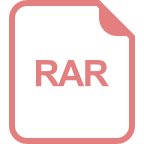
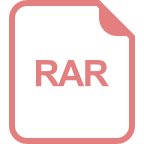
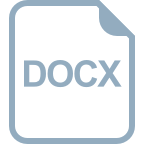
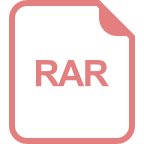
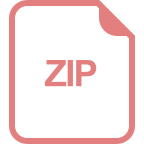
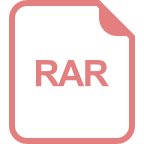
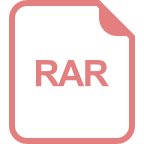
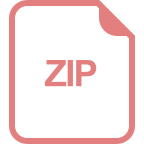
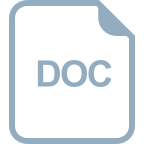
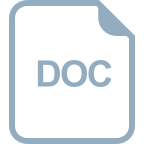
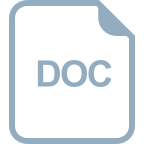
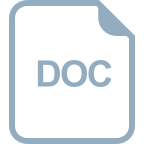
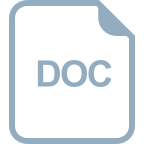
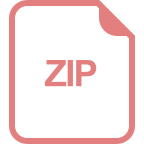