C++ 中如何把string中的每个字母都变成字母表中的下一位,如‘bz’变成‘ca’
时间: 2024-09-29 15:10:35 浏览: 16
在C++中,你可以使用ASCII码来实现这个功能。ASCII码是一种字符编码标准,其中每个字符都有对应的数字值。为了将字符串中的每个字母向后移动一位,你需要遍历字符串,检查当前字符是否是小写字母(ASCII码范围65到90对应大写字母,97到122对应小写字母),如果是,则加上1如果超过Z(或z)则减去26(因为字母表有26个字母)。下面是一个简单的示例:
```cpp
#include <iostream>
#include <cctype> // 包含tolower函数
std::string shiftLetters(const std::string& input) {
std::string result = "";
for (char c : input) {
if (islower(c)) { // 判断字符是否为小写字母
char shifted = static_cast<char>((c + 1 - 'a') % 26 + 'a'); // 向后移一位并保持在小写字母范围内
result += shifted;
} else if (isupper(c)) { // 对于大写字母,同样的逻辑但起点不同
char shifted = static_cast<char>((c + 1 - 'A') % 26 + 'A');
result += shifted;
} else {
result += c; // 非字母字符直接保留
}
}
return result;
}
int main() {
std::string str = "bz";
std::cout << shiftLetters(str) << std::endl; // 输出 "ca"
return 0;
}
```
相关推荐
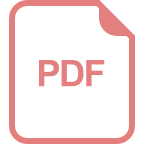
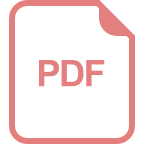
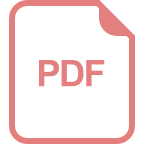
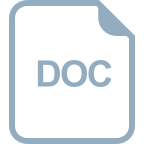
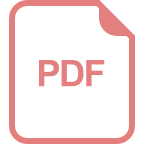
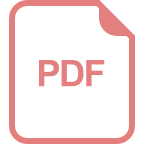
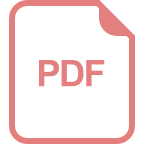
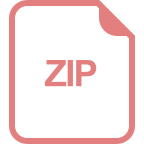
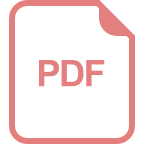
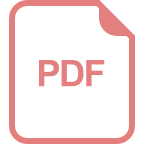
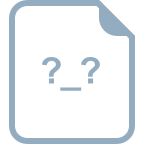
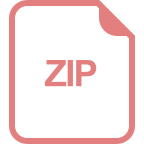