我要用opencacade创建一个立方体,并显示到QT窗口中,写一段代码,可能用到qt和vtk的相关代码,用c++
时间: 2024-09-24 16:28:25 浏览: 71
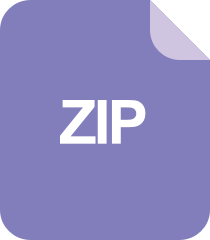
Qt-VTK-viewer:一个简单的 3D 视图嵌入 Qt 窗口的项目

为了在Qt中使用OpenCASCADE (OCCT) 创建一个立方体并在窗口中显示,你需要结合使用Qt GUI和OpenCASCADE的几何建模能力。这里是一个简化的示例,展示了如何在C++中实现这个功能:
```cpp
#include <QApplication>
#include <QMainWindow>
#include <QGraphicsView>
#include <QGraphicsScene>
#include <QGraphicsItem>
#include <TopoDS.hxx>
#include <BRepPrimAPI.hxx>
#include <GCPnts.hxx>
#include <gp_Vec.hxx>
#include <gp_Ax2.hxx>
#include <Qt3DCore/QEntity>
#include <Qt3DRender/QMeshRenderer>
#include <QOpenGLFunctions>
class Cube : public QObject {
public:
explicit Cube(QGraphicsScene* scene)
: m_scene(scene) {}
private Q_SLOTS:
void createCube() {
// 创建立方体
BRepPrimAPI_MakeBox box;
gp_Pnt center(0., 0., 0.);
gp_Dir xDir(1., 0., 0.), yDir(0., 1., 0.), zDir(0., 0., 1.);
box.SetBox(gp_Ax2(gp_Pnt(), xDir), gp_Ax2(gp_Pnt(), yDir), gp_Ax2(gp_Pnt(), zDir));
TopoDS_Shape shape = box.Shape();
TopoDS_Face face = TopoDS::Face(shape);
// 将几何转换为顶点数据
std::vector<QVector3D> vertices, normals;
for (const Handle(Geom_Surface)& surf : geomtools::SurfaceIterator(shape)) {
const Geom_LFT& lft = surf.LFT();
for (const Geom_Curve& curve : lft.Surfaces()) {
const Geom_Circle& circle = dynamic_cast<const Geom_Circle&>(curve);
const double radius = circle.Radius();
vertices.push_back(QVector3D(circle.Center().X(), circle.Center().Y(), circle.Center().Z()));
normals.push_back(QVector3D(circle.Axis().X(), circle.Axis().Y(), circle.Axis().Z()));
}
}
// 创建网格
auto mesh = new vtkSmartPointer<vtkPolyData>();
vtkSmartPointer<vtkPoints> points = vtkSmartPointer<vtkPoints>::New();
for (const QVector3D& vertex : vertices) {
points->InsertNextPoint(vertex.x(), vertex.y(), vertex.z());
}
mesh->SetPoints(points);
// 创建渲染器和网格材质
auto renderer = new QMeshRenderer();
renderer->setInput(mesh);
renderer->set shininess(32); // 设置光泽度
// 创建实体并添加到场景
auto entity = new Qt3DCore::QEntity(m_scene->rootItem());
entity->addComponent(renderer);
connect(this, &Cube::deleteLater, this, [entity] { delete entity; });
}
private:
QGraphicsScene* m_scene;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
QMainWindow window;
window.setCentralWidget(new QGraphicsView);
Cube cube(&window.scene());
connect(&cube, &QObject::destroyed, &a, &QApplication::quit);
cube.createCube();
window.show();
return a.exec();
}
```
这段代码首先创建了一个简单的Qt窗口和一个图形场景。然后它定义了一个名为`Cube`的类,该类负责创建立方体并将其渲染为一个`QMeshRenderer`。注意这里使用了VTK库(虽然未直接导入,因为它是Qt3D的一部分)来处理几何转换和网格创建。
阅读全文
相关推荐
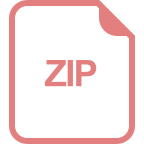
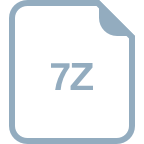
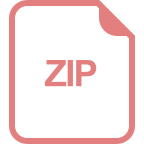
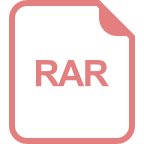
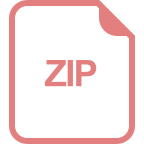
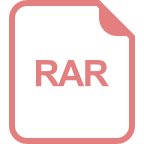
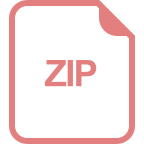
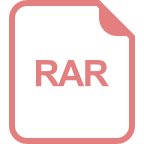
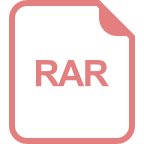
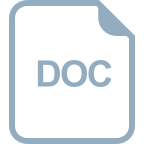
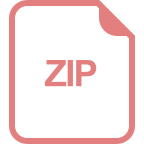
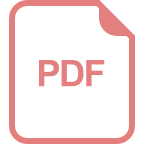
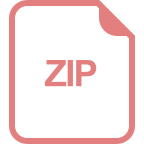
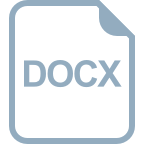