设计一个能细分为矩形、三角形、圆形和椭圆形的“图形”类。使用继承将这些图形分类找出能作为基类部分的共同特征(如校准点)和方法(如画法、初始化)并看看这些图形是否能进一步划分为子类。
时间: 2024-10-26 14:04:05 浏览: 63
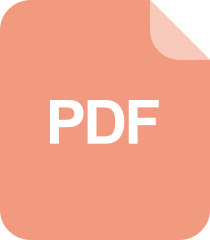
《三角形的分类》教案11.pdf,这是一份不错的文件
设计一个名为`Shape`的基类,它包含一些通用的属性和方法,比如坐标系上的位置(`x`, `y`)、大小、颜色等。同时,为了支持各种形状,我们可以添加如`draw()`这样的通用绘图方法:
```python
class Shape:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self):
pass # 简单的绘制函数,需要子类覆盖以实现具体形状
def set_position(self, x, y):
self.x = x
self.y = y
# 继承自Shape的子类
class Rectangle(Shape):
def __init__(self, x, y, width, height, color):
super().__init__(x, y, color)
self.width = width
self.height = height
def draw(self):
print(f"Drawing rectangle at ({self.x}, {self.y}) with size {self.width}x{self.height}")
class Triangle(Shape):
def __init__(self, x, y, base, height, color):
super().__init__(x, y, color)
self.base = base
self.height = height
def draw(self):
print(f"Drawing triangle at ({self.x}, {self.y}) with base {self.base} and height {self.height}")
class Circle(Shape):
def __init__(self, x, y, radius, color):
super().__init__(x, y, color)
self.radius = radius
def draw(self):
print(f"Drawing circle at ({self.x}, {self.y}) with radius {self.radius}")
class Ellipse(Shape):
def __init__(self, x, y, major_radius, minor_radius, color):
super().__init__(x, y, color)
self.major_radius = major_radius
self.minor_radius = minor_radius
def draw(self):
print(f"Drawing ellipse at ({self.x}, {self.y}) with major radius {self.major_radius} and minor radius {self.minor_radius}")
```
在这个设计中,`Shape`类包含了所有图形的基本属性和共性的行为,如位置和颜色设置。每个具体的形状(如Rectangle、Triangle、Circle和Ellipse)都通过继承`Shape`,并重写`draw`方法来体现它们的独特性质。
阅读全文
相关推荐
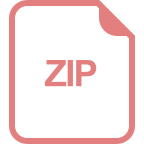
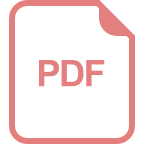



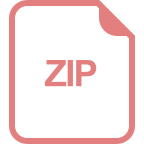
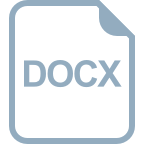
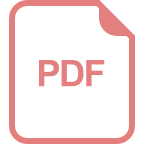
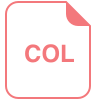
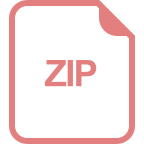
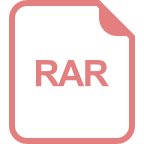