使用postAtTime, Calendar, runnable实现在每天晚上6点自动调用runnable
时间: 2024-09-13 19:05:48 浏览: 35
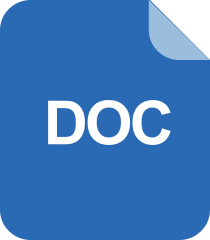
Android学习笔记(三一):线程:Message和Runnable.doc
在Java中,可以使用`ScheduledExecutorService`类结合`postAtTime`方法和`Calendar`对象来实现定时任务。具体来说,你可以使用`postAtTime`方法来安排一个`Runnable`任务在指定的时间执行。以下是一个简单的例子,展示如何设置一个任务,使其每天晚上6点执行:
```java
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
import java.util.Calendar;
public class DailyTaskScheduler {
public static void main(String[] args) {
// 创建一个ScheduledExecutorService实例
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
// 创建一个Runnable任务
Runnable task = new Runnable() {
@Override
public void run() {
// 这里填写需要执行的任务代码
System.out.println("任务执行了!");
}
};
// 获取当前时间的Calendar实例
Calendar calendar = Calendar.getInstance();
// 设置为今天的晚上6点
calendar.set(Calendar.HOUR_OF_DAY, 18);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MILLISECOND, 0);
// 如果当前时间已经超过今天6点,则设置为明天的6点
if (calendar.before(Calendar.getInstance())) {
calendar.add(Calendar.DATE, 1);
}
// 计算第一次执行任务的延迟时间
long initialDelay = calendar.getTimeInMillis() - System.currentTimeMillis();
// 使用postAtTime方法安排任务在每天的6点执行
scheduler.scheduleAtFixedRate(task, initialDelay, TimeUnit.DAYS.toMillis(1), TimeUnit.MILLISECONDS);
}
}
```
在这个例子中,首先创建了一个`ScheduledExecutorService`实例,然后定义了一个`Runnable`任务。通过`Calendar`对象获取当前时间,并设置为当天晚上6点。如果当前时间已经超过了今天的6点,那么设置为明天的6点。然后计算出需要等待的时间(延迟时间),使用`scheduleAtFixedRate`方法来安排任务,使其每24小时执行一次。
注意,上面的代码中使用了`scheduleAtFixedRate`方法,而不是`postAtTime`方法。`postAtTime`通常用于`ScheduledExecutorService`的`ScheduledFuture`对象来重新调度任务,而不是直接在`ScheduledExecutorService`中使用。上面的代码展示了如何实现定时任务,没有直接使用`postAtTime`方法。
阅读全文
相关推荐
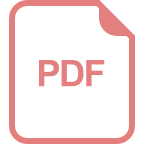
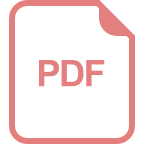






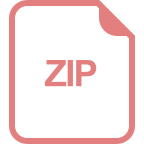
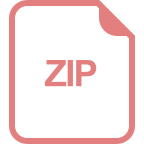