个Stude创建一nt类 ,编写方法public StudentInfo[] inputStuInfo(),通过键盘分别输入三个学生的学号、姓名、班级信息存入StudentInfo类型的数组并返回。
时间: 2025-01-01 11:39:01 浏览: 6
在Java中,你可以创建一个名为`Student`的类,并在其中定义一个名为`inputStuInfo`的方法,这个方法会从用户那里获取三个学生的学号、姓名和班级信息,并将这些数据存储在一个`StudentInfo`类型的数组中。这里假设`StudentInfo`是一个包含学号、姓名和班级属性的简单类。
```java
import java.util.Scanner;
class Student {
// 定义一个静态内部类 StudentInfo,表示学生的信息结构
static class StudentInfo {
int studentId;
String name;
String className;
public StudentInfo(int studentId, String name, String className) {
this.studentId = studentId;
this.name = name;
this.className = className;
}
}
// 方法输入学生信息并返回StudentInfo数组
public static StudentInfo[] inputStuInfo() {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入第一个学生的学号:");
int id1 = scanner.nextInt();
System.out.println("请输入第一个学生的姓名:");
String name1 = scanner.nextLine(); // 使用nextLine()避免回车字符
System.out.println("请输入第一个学生的班级:");
String className1 = scanner.nextLine();
System.out.println("请输入第二个学生的学号:");
int id2 = scanner.nextInt();
// ... (类似地获取第二、第三个学生的相关信息)
// 创建并初始化数组,假设最多只有三个学生
StudentInfo[] students = new StudentInfo[3];
students[0] = new StudentInfo(id1, name1, className1);
students[1] = new StudentInfo(scanner.nextInt(), scanner.nextLine(), scanner.nextLine());
if (students.length > 1) { // 如果还有空位,继续获取信息
students[2] = new StudentInfo(scanner.nextInt(), scanner.nextLine(), scanner.nextLine());
}
return students;
}
// 主函数示例
public static void main(String[] args) {
StudentInfo[] stuInfos = inputStuInfo();
for (StudentInfo info : stuInfos) {
System.out.printf("学号:%d, 姓名:%s, 班级:%s%n", info.studentId, info.name, info.className);
}
}
}
```
阅读全文
相关推荐





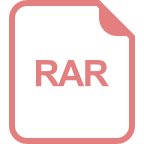












