迷宫游戏python源码
时间: 2023-07-24 17:01:54 浏览: 196
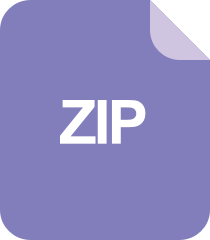
基于Q-Learning强化学习算法走迷宫游戏python源码.zip
### 回答1:
迷宫游戏是一个经典的游戏,玩家需要在迷宫中寻找出口。下面是一个使用Python编写的迷宫游戏源码:
```python
import random
# 定义迷宫类
class Maze:
def __init__(self, rows, cols):
self.rows = rows
self.cols = cols
self.maze = []
self.generate_maze()
# 生成迷宫
def generate_maze(self):
self.maze = [['#'] * self.cols for _ in range(self.rows)]
stack = [(0, 0)]
while stack:
x, y = stack[-1]
self.maze[x][y] = ' '
# 获取随机邻居
neighbors = []
if x > 1 and self.maze[x-2][y] == '#':
neighbors.append((x-2, y))
if x < self.rows-2 and self.maze[x+2][y] == '#':
neighbors.append((x+2, y))
if y > 1 and self.maze[x][y-2] == '#':
neighbors.append((x, y-2))
if y < self.cols-2 and self.maze[x][y+2] == '#':
neighbors.append((x, y+2))
if neighbors:
nx, ny = random.choice(neighbors)
self.maze[(nx+x)//2][(ny+y)//2] = ' '
stack.append((nx, ny))
else:
stack.pop()
# 打印迷宫
def print_maze(self):
for row in self.maze:
print(' '.join(row))
# 创建迷宫对象并打印迷宫
maze = Maze(11, 11)
maze.print_maze()
```
此源码使用递归回溯算法生成一个随机迷宫,并通过二维列表来表示迷宫的格子。迷宫的入口是左上角的位置(0, 0),出口位置则是随机生成的。每个方块可以是墙壁(#)或通路(空格),玩家需要通过移动来找到出口。
### 回答2:
迷宫游戏是一种常见的游戏,在Python中可以使用代码来实现。下面是一个简单的迷宫游戏的Python源码示例:
```python
import numpy as np
# 迷宫地图
maze = np.array([[1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 1, 0, 0, 0, 1, 1],
[1, 0, 0, 0, 1, 0, 1, 1],
[1, 1, 1, 0, 1, 0, 0, 1],
[1, 0, 0, 0, 0, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1]])
# 设置起点和终点
start = (1, 1)
end = (6, 6)
# 创建一个空迷宫路径记录
path = np.zeros_like(maze)
# 定义一个递归函数来找到路径
def find_path(maze, start, end, path):
# 如果当前位置是终点,则找到路径
if start == end:
return True
# 如果当前位置超出迷宫范围或者是墙壁,则返回False
if maze[start] != 0:
return False
# 标记当前位置已访问
path[start] = 1
# 递归查找四个方向上的路径
if find_path(maze, (start[0]+1, start[1]), end, path) or \
find_path(maze, (start[0]-1, start[1]), end, path) or \
find_path(maze, (start[0], start[1]+1), end, path) or \
find_path(maze, (start[0], start[1]-1), end, path):
return True
# 如果四个方向上都没有路径,则返回False
return False
# 查找并打印路径
if find_path(maze, start, end, path):
print('找到路径:')
print(path)
else:
print('未找到路径。')
```
这段代码使用了递归算法来查找迷宫中的路径。迷宫地图使用二维数组表示,其中1表示墙壁,0表示可通过的路径。起点和终点分别指定为`(1, 1)`和`(6, 6)`。使用`find_path`函数来递归查找路径,如果找到路径则返回True,否则返回False。最后,根据查找结果打印相应的信息。
请注意,这只是一个简单的示例代码,实际迷宫游戏可能还包括更复杂的功能,例如计时、计分等。
阅读全文
相关推荐
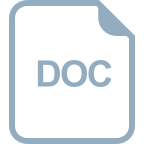
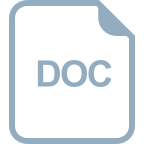
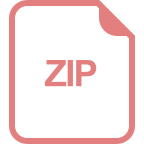
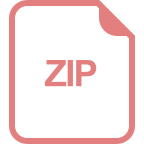
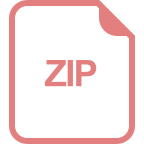
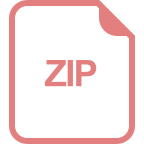
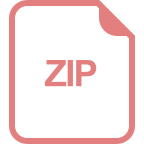
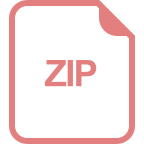
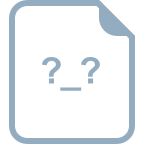
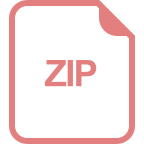
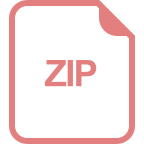
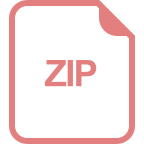
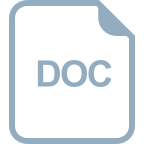
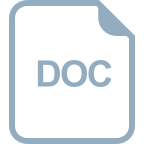
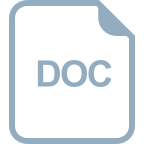
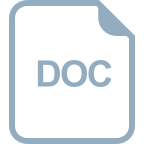
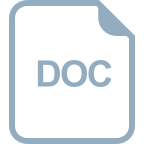
