基于MyBatis框架XML式开发,设计实现一个通讯录管理系统。
时间: 2024-11-09 11:25:58 浏览: 3
在基于MyBatis的XML式开发中,我们可以构建一个通讯录管理系统,主要包括以下几个步骤:
1. **数据库设计**:首先设计数据库结构,比如用户表(User)、联系人表(Contact),包含字段如用户ID、姓名、电话等。
```sql
CREATE TABLE User (
id INT PRIMARY KEY,
name VARCHAR(50),
password VARCHAR(100)
);
CREATE TABLE Contact (
id INT PRIMARY KEY,
user_id INT,
contact_name VARCHAR(50),
phone VARCHAR(20),
FOREIGN KEY (user_id) REFERENCES User(id)
);
```
2. **Mapper接口编写**:创建UserMapper和ContactMapper接口,用于定义SQL操作。
```java
public interface UserMapper {
@Select("SELECT * FROM User WHERE id = #{id}")
User getUserById(int id);
@Insert("INSERT INTO User(name, password) VALUES(#{name}, #{password})")
int createUser(User user);
}
public interface ContactMapper {
@Select("SELECT * FROM Contact WHERE user_id = #{userId}")
List<Contact> getContactsByUserId(int userId);
@Insert("INSERT INTO Contact(user_id, contact_name, phone) VALUES(#{userId}, #{contactName}, #{phone})")
int addContact(Contact contact);
}
```
3. **XML映射文件**:在resources/mapper目录下创建对应的xml文件,实现Mapper接口的方法到具体的SQL查询。
```xml
<mapper namespace="com.example.UserMapper">
<select id="getUserById" resultType="com.example.User">
SELECT * FROM User WHERE id = #{id}
</select>
</mapper>
<mapper namespace="com.example.ContactMapper">
<select id="getContactsByUserId" resultType="com.example.Contact">
SELECT * FROM Contact WHERE user_id = #{userId}
</select>
<insert id="addContact">
INSERT INTO Contact(user_id, contact_name, phone) VALUES(#{userId}, #{contactName}, #{phone})
</insert>
</mapper>
```
4. **Service层处理**:在业务层(Service层)中,通过SqlSession从数据库获取数据并完成业务逻辑,例如保存用户和联系人。
```java
@Service
public class UserService {
private SqlSessionFactory sqlSessionFactory;
public void addUser(User user) {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
int result = mapper.createUser(user);
// 数据库操作后处理...
} finally {
sqlSession.close();
}
}
public List<Contact> getContactsForUser(int userId) {
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
ContactMapper mapper = sqlSession.getMapper(ContactMapper.class);
return mapper.getContactsByUserId(userId);
} finally {
sqlSession.close();
}
}
}
```
5. **UI展示**:最后在前端页面上展示用户信息和联系人列表,并通过Controller层调用Service层的方法获取数据。
阅读全文
相关推荐
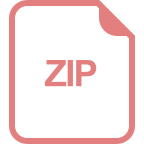
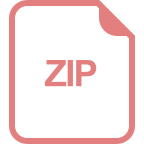
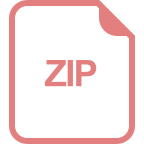

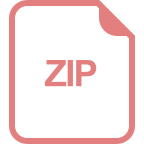
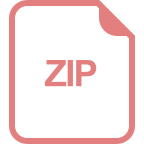
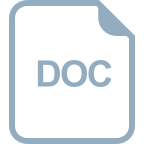
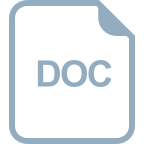
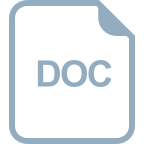
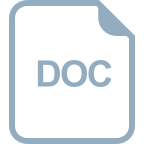
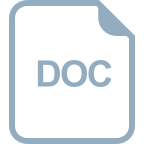
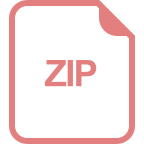
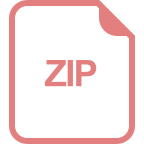