C# 串口高速通信下解决数据处理慢 代码
时间: 2024-09-13 12:13:26 浏览: 100
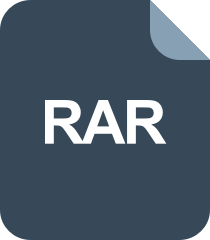
C#上位机开发-串口通信,读取并处理数据
在C#中进行串口高速通信时,如果发现数据处理速度慢,可能是由于在接收数据时使用了阻塞模式,或者是在处理数据时采用了不当的方式。为了优化数据处理速度,可以考虑以下几个方面:
1. 使用异步通信:避免在主线程中直接处理串口数据,而是使用异步模式接收数据,这样可以避免阻塞主线程,提高程序的响应性。
2. 使用缓冲区:合理设置接收缓冲区的大小,确保能够快速有效地接收数据。使用循环缓冲区或队列来管理接收到的数据,防止数据溢出。
3. 数据处理分离:将数据接收和数据处理分离到不同的线程中,这样可以避免处理数据时阻塞接收数据的线程。
4. 使用内存流(MemoryStream):当需要处理大量串口数据时,可以先将数据读入内存流中,再从中读取和处理数据,这样可以提高数据处理的效率。
以下是一个简化的C#代码示例,展示了如何使用异步模式进行串口通信并使用内存流处理数据:
```csharp
using System;
using System.IO.Ports;
using System.Threading.Tasks;
using System.Threading;
public class SerialPortHighSpeedCommunication
{
private SerialPort serialPort;
private MemoryStream memoryStream = new MemoryStream();
public SerialPortHighSpeedCommunication(string portName, int baudRate)
{
serialPort = new SerialPort(portName, baudRate);
serialPort.DataReceived += OnDataReceived;
}
private void OnDataReceived(object sender, SerialDataReceivedEventArgs e)
{
try
{
int bytesToRead = serialPort.BytesToRead;
byte[] buffer = new byte[bytesToRead];
int bytesRead = serialPort.Read(buffer, 0, bytesToRead);
// 将接收到的数据写入内存流
memoryStream.Write(buffer, 0, bytesRead);
}
catch (Exception ex)
{
Console.WriteLine($"Error: {ex.Message}");
}
}
public async Task ProcessDataAsync(CancellationToken cancellationToken)
{
// 使用异步流处理内存流中的数据
await foreach (var data in ProcessDataStreamAsync(cancellationToken))
{
// 处理数据
Console.WriteLine($"Processed data: {data}");
}
}
private async IAsyncEnumerable<byte[]> ProcessDataStreamAsync(CancellationToken cancellationToken)
{
memoryStream.Position = 0; // 重置内存流位置
byte[] buffer = new byte[1024]; // 设置单次处理的数据大小
while (!cancellationToken.IsCancellationRequested)
{
int bytesRead = await memoryStream.ReadAsync(buffer, cancellationToken);
if (bytesRead > 0)
{
yield return buffer.Take(bytesRead).ToArray();
}
}
}
public void Start()
{
if (!serialPort.IsOpen)
{
serialPort.Open();
}
}
public void Stop()
{
if (serialPort.IsOpen)
{
serialPort.Close();
}
}
}
// 使用示例
public static async Task Main(string[] args)
{
SerialPortHighSpeedCommunication comPort = new SerialPortHighSpeedCommunication("COM3", 9600);
comPort.Start();
CancellationTokenSource cts = new CancellationTokenSource();
Task processingTask = comPort.ProcessDataAsync(cts.Token);
Console.WriteLine("Press any key to stop...");
Console.ReadKey();
cts.Cancel();
await processingTask;
comPort.Stop();
}
```
在这个示例中,我们创建了一个`SerialPortHighSpeedCommunication`类,它使用异步模式接收串口数据,并使用内存流暂存接收到的数据。数据处理函数`ProcessDataAsync`是一个异步函数,它通过`ProcessDataStreamAsync`异步流来处理内存流中的数据。
阅读全文
相关推荐
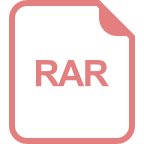
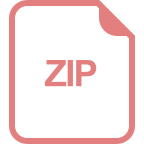
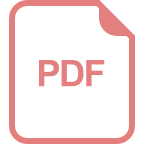
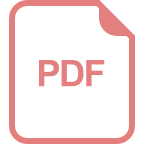
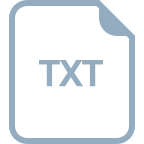
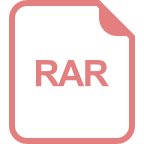
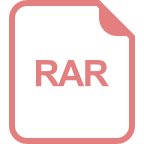
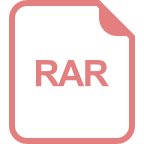
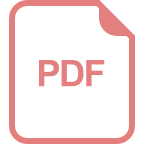
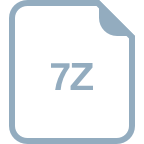
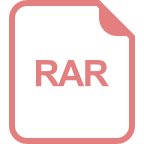
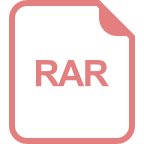
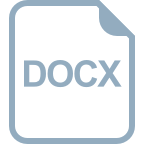
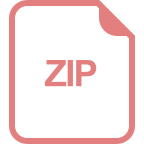
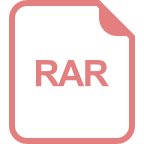
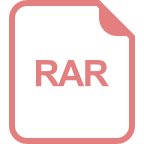
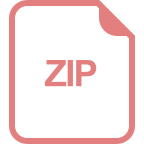
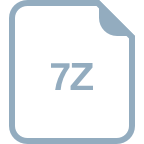