C语言从键盘输入一串英文单词和数字,以@结束,统计输入的大写字母、小写字母、数字和空格的字符数
时间: 2024-11-22 07:38:58 浏览: 29
在C语言中,你可以通过以下步骤来完成这个任务:
1. 首先,创建一个循环,让用户持续输入直到遇到`@`为止。
2. 对于每个输入的字符,检查它的ASCII值:
- 如果是大写字母(ASCII值在65到90之间),增加大写字母计数。
- 如果是小写字母(ASCII值在97到122之间),增加小写字母计数。
- 如果是数字(ASCII值在48到57之间),增加数字计数。
- 如果是空格(ASCII值为32),增加空格计数。
3. 当遇到`@`时,跳出循环,并打印出统计结果。
以下是简单的示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int uppercase = 0, lowercase = 0, digits = 0, spaces = 0;
char input;
while ((input = getchar()) != '@') { // 读取用户输入直到遇见@
if (isupper(input)) { // 检查是否为大写字母
uppercase++;
} else if (islower(input)) { // 检查是否为小写字母
lowercase++;
} else if (isdigit(input)) { // 检查是否为数字
digits++;
} else if (isspace(input)) { // 检查是否为空格
spaces++;
}
}
printf("Uppercase letters: %d\n", uppercase);
printf("Lowercase letters: %d\n", lowercase);
printf("Digits: %d\n", digits);
printf("Spaces: %d\n", spaces);
return 0;
}
```
当你运行这段程序并输入一些文字和数字,然后按`@`结束时,它会统计并显示每种类型的字符数量。
阅读全文
相关推荐
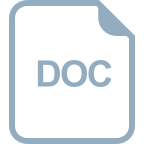
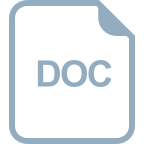
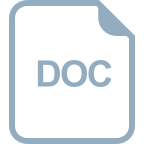















