至少使用3种方法模拟数据下载,使用progressBar组件,AsyncTask类现在不要使用
时间: 2024-10-25 17:13:58 浏览: 22
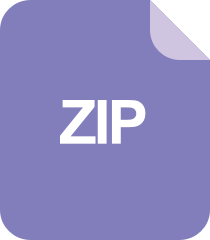
Android中Intent和ProgressBar的结合使用
模拟数据下载并展示进度条通常涉及到网络请求、进度更新和UI同步。这里提供三种非AsyncTask的方式来实现这个功能:
1. **线程+Handler**: 使用`Thread`发起网络请求,并通过`Handler`在主线程中更新UI元素,如`ProgressBar`。创建一个自定义`Handler`用于传递下载进度信息。
```java
new Thread(new Runnable() {
@Override
public void run() {
// 模拟下载过程
int totalSize = getTotalFileSize();
for (int i = 0; i < totalSize; i++) {
int progress = (i * 100) / totalSize;
Handler handler = new Handler(Looper.getMainLooper());
handler.post(() -> progressBar.setProgress(progress));
// 这里处理网络请求和下载逻辑
}
}
}).start();
```
2. **LiveData + ViewModel**: 如果你在使用Android Architecture Components,可以使用`LiveData`和`ViewModel`来管理下载状态。每次下载进度改变时,更新`LiveData`值,然后在观察者(通常是`RecyclerView`的Adapter或Fragment界面)中更新UI。
```java
// ViewModel
MutableLiveData<Integer> downloadProgress = new MutableLiveData<>();
...
void startDownload(int totalSize) {
// 开始下载
for (int i = 0; i < totalSize; i++) {
int progress = (i * 100) / totalSize;
downloadProgress.postValue(progress);
}
}
// UI
downloadProgress.observe(this, progress -> progressBar.setProgress(progress));
```
3. **Kotlin Coroutines**: 利用协程(Coroutines)可以在IO密集型任务中轻松地并发处理,并使用`withContext`确保UI更新在主线程执行。例如,你可以创建一个CoroutineScope,配合`flow`发布进度变化。
```kotlin
val scope = CoroutineScope(Dispatchers.Main)
scope.launch {
val totalSize = getTotalFileSize()
for (i in 0 until totalSize) {
val progress = (i * 100) / totalSize
withContext(Dispatchers.Main) {
progressBar.setProgress(progress)
}
// 模拟下载操作
}
}
```
阅读全文
相关推荐
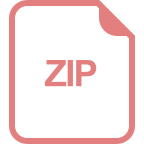
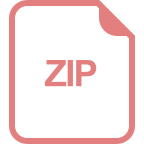

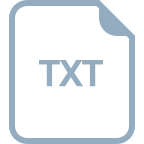
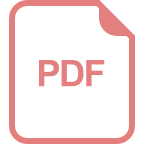
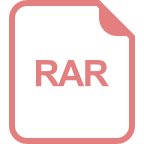
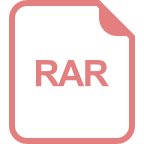
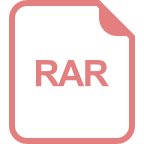
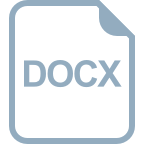
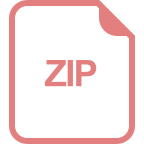
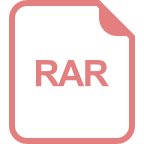
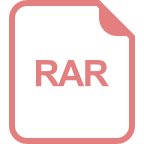
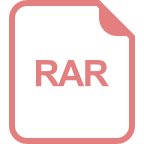
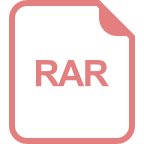
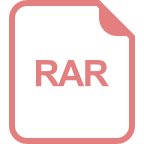
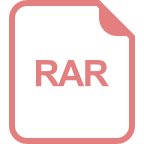
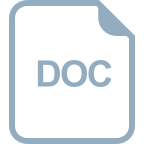