小明拿50元钱到某商店换零钱。假设商店只有面值为1元、2元和5元的钞票,且每种钞票的数量都足够多。最后换给了小明20张钞票,问有多少种换法?输出每种换法中各种面额钞票的张数。(C语言实现)
时间: 2024-12-16 16:27:05 浏览: 19
这是一个经典的组合问题,可以用动态规划或回溯算法来解决。在这里,我们使用动态规划来计算所有可能的组合。为了表示每种换法,我们可以创建一个数组`dp[i]`,其中`i`是从0到49的整数,表示有`i`元的钱需要找零,`dp[i]`存储的是对应金额找零所需的最小张数。
下面是使用C语言的简单实现:
```c
#include <stdio.h>
#define MAX_MONEY 50
#define NUM_BILLS (3) // 1元、2元、5元三种面额
int dp[MAX_MONEY + 1]; // 初始化为正无穷大,因为找不到钱的情况
int bill_count[NUM_BILLS] = {0}; // 记录每种面额的张数
void find_combinations(int money_left, int index) {
if (money_left == 0) {
for (int i = 0; i < NUM_BILLS; i++) {
printf("%d元%d张 ", bill_count[i], i);
}
printf("\n");
return;
}
// 如果当前面额大于剩余金额,跳过
if (index >= NUM_BILLS || bill_count[index] > money_left / index) {
return;
}
// 不取这个面额
dp[money_left] = dp[money_left - index * bill_count[index]];
bill_count[index]++;
// 取这个面额
dp[money_left] = min(dp[money_left], dp[money_left - index * bill_count[index]] + 1);
bill_count[index]--;
// 深度优先搜索剩下的金额
find_combinations(money_left, index);
}
int main() {
dp[0] = 0; // 0元不需要找零
for (int i = 1; i <= 50; i++) {
dp[i] = MAX_MONEY; // 初始化其他情况尽可能多的找零张数
}
find_combinations(50, 0);
return 0;
}
```
当你运行这个程序,它会输出所有可能的找零方案及其对应的钞票张数。注意,这将输出所有可能的解,并非只求最小张数。对于这个问题,由于枚举的复杂性,实际应用中可能会使用更高效的算法优化。
阅读全文
相关推荐
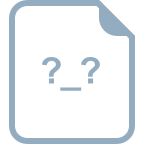
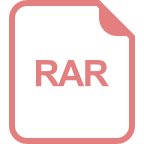








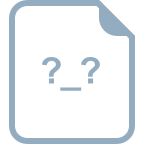



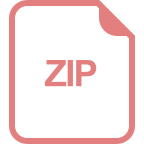
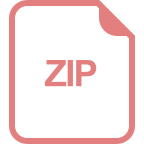
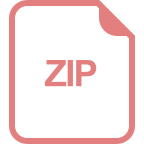
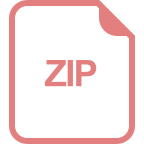