Vue.js跳转页面
时间: 2023-12-27 07:23:49 浏览: 27
Vue.js提供了多种方式实现页面跳转,以下是其中的两种方式:
1.使用`this.$router.push()`方法进行跳转:
```javascript
// 在methods中定义跳转方法
methods: {
goHome() {
this.$router.push("/"); // 跳转到主页
},
goLogin() {
this.$router.push("/login"); // 跳转到登录页
}
}
```
2.使用`<router-link>`标签进行跳转:
```html
<!-- 在模板中使用<router-link>标签 -->
<router-link to="/">跳转到主页</router-link>
<router-link to="/login">跳转到登录页</router-link>
```
相关问题
vue.js实现页面跳转
Vue.js 是一个前端框架,可以通过路由实现页面跳转。
首先,需要在项目中安装 `vue-router`,可以使用 npm 命令进行安装:
```
npm install vue-router
```
然后,在 Vue.js 应用程序的入口文件中,引入 `vue-router` 并使用它:
```
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter)
const router = new VueRouter({
routes: [
{
path: '/home',
component: Home
},
{
path: '/about',
component: About
}
]
})
new Vue({
router,
render: h => h(App),
}).$mount('#app')
```
在路由配置中,我们定义了两个路由 `/home` 和 `/about`,并指定了对应的组件。然后将路由实例注入到 Vue 实例中,这样就能在应用程序中使用路由了。
在组件中,可以使用 `router-link` 标签来实现页面跳转,例如:
```
<template>
<div>
<router-link to="/home">Home</router-link>
<router-link to="/about">About</router-link>
</div>
</template>
```
当用户点击链接时,路由会自动根据配置进行跳转。同时,也可以使用 `this.$router.push()` 方法来进行编程式跳转,例如:
```
methods: {
goToHome() {
this.$router.push('/home')
}
}
```
vue.js 实现点击按钮跳转页面
要实现点击按钮跳转页面,可以使用 Vue.js 提供的路由功能。首先需要在 Vue.js 项目中配置路由,然后在按钮的点击事件中通过编程方式跳转到指定的页面。
以下是实现的具体步骤:
1. 配置路由
在 Vue.js 项目的 router/index.js 文件中定义路由:
```
import Vue from 'vue'
import Router from 'vue-router'
import HomePage from '@/components/HomePage'
import OtherPage from '@/components/OtherPage'
Vue.use(Router)
export default new Router({
routes: [
{
path: '/',
name: 'HomePage',
component: HomePage
},
{
path: '/other',
name: 'OtherPage',
component: OtherPage
}
]
})
```
上面的代码定义了两个路由,分别对应首页和其他页面。
2. 在按钮点击事件中跳转页面
在 Vue.js 组件的 methods 中定义按钮的点击事件,并且使用 Vue.js 提供的 $router.push() 方法跳转到指定的页面:
```
<template>
<div>
<button @click="goToOtherPage">跳转到其他页面</button>
</div>
</template>
<script>
export default {
methods: {
goToOtherPage () {
this.$router.push('/other')
}
}
}
</script>
```
上面的代码中,通过在按钮的 click 事件中调用 goToOtherPage 方法来跳转到指定的页面。在方法中使用 this.$router.push() 方法并传入路由路径来实现页面跳转。
以上就是使用 Vue.js 实现点击按钮跳转页面的基本方法。
相关推荐
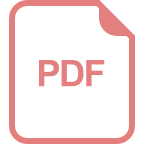
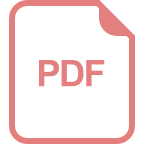
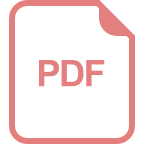
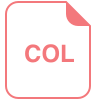
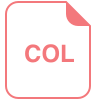
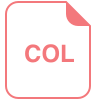
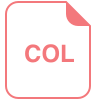
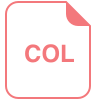







