数据结构与算法c语言 编写一个程序,读文件file1.txt的内容,例如“12 34 56”,逆序输出到file2.txt中,例如“56 34 12”.
时间: 2024-09-18 17:00:40 浏览: 60
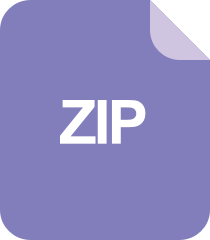
link,c语言文件批量移动工具源码,c语言程序
在C语言中,我们可以使用标准库函数`fopen`, `fgets`, 和`fprintf`来实现这个功能。首先打开输入文件,然后逐行读取内容,分割字符串为整数数组,接着反转数组,最后将逆序后的数字写入到输出文件。这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 函数用于按空格分割字符串并存入整数数组
void split_string(char *str, int arr[], size_t len) {
char *token = strtok(str, " ");
for (size_t i = 0; token && i < len; ++i, token = strtok(NULL, " ")) {
arr[i] = atoi(token);
}
}
// 函数用于反转整数数组
void reverse_array(int arr[], size_t len) {
for (size_t i = 0, j = len - 1; i < j; ++i, --j) {
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int main() {
FILE *input_file = fopen("file1.txt", "r");
if (!input_file) {
perror("Error opening input file");
return 1;
}
FILE *output_file = fopen("file2.txt", "w");
if (!output_file) {
perror("Error opening output file");
fclose(input_file);
return 1;
}
// 读取文件内容,并处理每一行
char line[100];
while (fgets(line, sizeof(line), input_file)) {
size_t len = strlen(line);
if (line[len - 1] == '\n') { // 去除换行符
line[len - 1] = '\0';
}
int numbers[10]; // 假设一行最多有10个数字
split_string(line, numbers, sizeof(numbers));
reverse_array(numbers, sizeof(numbers) / sizeof(numbers[0]));
// 将逆序后的数字写回文件
for (size_t i = 0; i < sizeof(numbers) / sizeof(numbers[0]); ++i) {
fprintf(output_file, "%d ", numbers[i]);
}
fprintf(output_file, "\n"); // 每行结束添加换行符
}
fclose(input_file);
fclose(output_file);
return 0;
}
```
运行此程序后,它会读取`file1.txt`的内容,逆序处理后再写入到`file2.txt`。
阅读全文
相关推荐
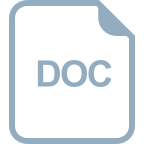
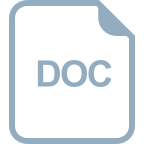
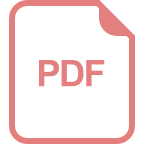
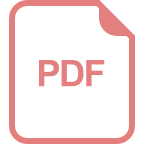
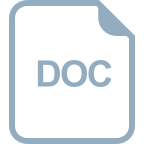
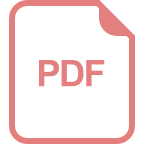
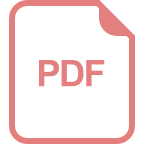
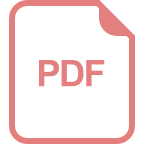
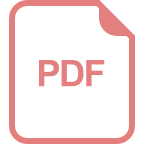
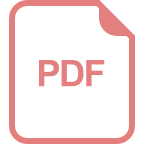
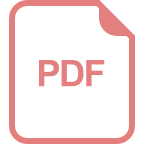
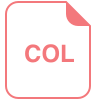
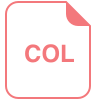
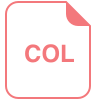
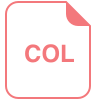


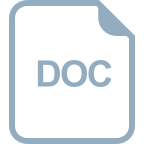