上例中的scr.jpg图像用程序实现打开,分别显示该图像的R通道颜色、B通道颜色。然后将该图像转化为灰度图像并显示
时间: 2024-12-17 14:47:38 浏览: 11
在Python中,我们可以使用PIL(Pillow库)来处理图像文件,包括读取、通道操作和转换为灰度图。这里是一个简单的例子:
首先,确保已经安装了`Pillow`库,如果没有,可以使用`pip install pillow`进行安装。
```python
from PIL import Image
# 打开 'scr.jpg' 图像文件
image = Image.open('scr.jpg')
# 分离图像的 R、G、B 通道
r_channel = image.convert('L') # R通道即为灰度通道
b_channel = image.split()[0] # B通道直接通过split()方法获取
# 显示 R 和 B 通道的颜色
# 因为 R 通道已经是灰度,可以直接显示
r_channel.show(title="R Channel")
# B 通道需要先转换回灰度再显示
b_grayscale = b_channel.convert('L')
b_grayscale.show(title="B Channel (Grayscale)")
# 将原图转为灰度
gray_image = image.convert('L')
gray_image.show(title="Original Image in Grayscale")
```
上述代码会依次打开图片,显示R通道的灰度图像,然后显示原始B通道(需要转换成灰度),最后显示整个图像的灰度版本。
相关问题
通过python程序实现打开该图像并显示图像和图像的形状(行,列,深度),最后将该图像保存为“scr.jpg”格式文件。
在Python中,你可以使用PIL(Python Imaging Library)或其后续项目Pillow库来处理图片的读取、展示和保存。以下是简单的步骤:
首先,你需要安装Pillow库,如果你还没有安装,可以使用pip安装:
```bash
pip install pillow
```
然后,你可以编写如下的代码来实现你的需求:
```python
from PIL import Image
# 打开图像文件
image_path = "your_image_file.jpg" # 替换为你的图片路径
with Image.open(image_path) as img:
# 显示图像
img.show()
# 获取图像的尺寸(行,列)
width, height = img.size
print(f"图像的尺寸为:{width}x{height}")
# 检查图像是否有深度(通常是RGB,通道数为3)
if len(img.getbands()) == 3:
depth = 3
print(f"图像有3个颜色通道,即深度为3")
elif len(img.getbands()) == 4:
depth = 4
print(f"图像有4个颜色通道,包括一个透明度通道")
else:
depth = len(img.getbands())
print(f"图像有{depth}个颜色通道")
# 保存图像到新的文件
new_path = "scr.jpg"
img.save(new_path)
print(f"图像已保存至'{new_path}'.")
scr = cv2.imread('first.jpg') scr = cv2.cv2tColor(scr, cv2.COLOR_BGR2GRAY) #转换为灰度图像 dft = cv2.dft(np.float32(scr), flags=cv2.DFT_COMPLEX_OUTPUT) #傅里叶变换 dft_shift = np.fft.fftshift(dft) # 零频域移到频谱中心 rows, cols = scr.shape[:2] sigma2 = 0.01 x, y = np.mgrid[-1:1:2.0 / rows, -1:1:2.0 / cols] z = 1 / (2 * np.pi * sigma2) * np.exp(-(x ** 2 + y ** 2) / (2 * sigma2)) zNorm = np.uint8(cv2.normalize(z, None, 0, 255, cv2.NORM_MINMAX)) # 归一化为 [0,255] maskGauss = np.zeros((rows, cols, 2), np.uint8) maskGauss[:, :, 0] = zNorm maskGauss[:, :, 1] = zNorm fshift = dft_shift * maskGauss # mask与频域进行点乘 f_ishift = np.fft.ifftshift(fshift) # 将零频域移到左上角 img_back = cv2.idft(f_ishift) # 转换为空间域 img_back = cv2.magnitude(img_back[:, :, 0], img_back[:, :, 1]) max = np.max(img_back) min = np.min(img_back) res = np.zeros((rows, cols), dtype="uint8") # 高斯滤波结果 for i in range(rows): for j in range(cols): res[i, j] = 255 * (img_back[i, j] - min) / (max - min) #归一化为[0, 255]
这段代码的作用是对一张图片进行高斯滤波。首先,使用OpenCV库中的cv2.imread()函数读取图片,然后使用cv2.cvtColor()函数将图片转换为灰度图像。接着,使用cv2.dft()函数对灰度图像进行傅里叶变换,将傅里叶变换结果移动到频谱中心。然后,定义一个高斯滤波器,使用np.mgrid()函数生成高斯滤波器的权重矩阵z。该矩阵归一化后作为mask与频域进行点乘,得到fshift。使用np.fft.ifftshift()函数将零频域移到左上角,使用cv2.idft()函数将f_ishift转换为空间域。最后,将结果归一化到[0, 255]范围内。
阅读全文
相关推荐
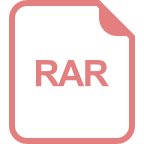
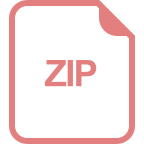
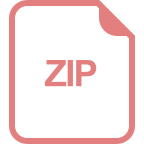
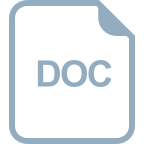

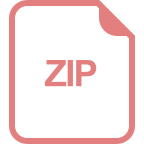
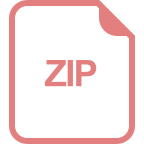
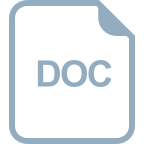
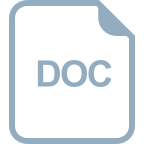
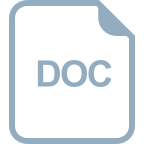
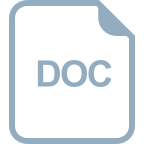






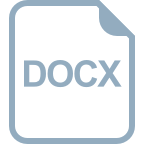