如果我想用opencv-python来进行红色操场车道线的检测,请写出具体有效的代码
时间: 2024-11-22 15:38:26 浏览: 8
在Python中使用OpenCV库进行红色车道线检测,通常需要经过以下几个步骤:
1. 导入必要的库:
```python
import cv2
import numpy as np
```
2. 加载图像并转换到HSV色彩空间,因为颜色的分离在HSV更容易进行:
```python
image = cv2.imread('lane_image.jpg')
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
```
3. 定义红色的HSV范围,这里假设红色大约在(0, 50, 50)到(10, 255, 255)之间,并排除一些噪声值:
```python
lower_red = np.array([0, 50, 50])
upper_red = np.array([10, 255, 255])
```
4. 创建一个红色的掩码:
```python
mask = cv2.inRange(hsv_image, lower_red, upper_red)
```
5. 对掩码进行二值化处理,以便于后续边缘检测:
```python
_, binary_mask = cv2.threshold(mask, 127, 255, cv2.THRESH_BINARY)
```
6. 使用`cv2.HoughLinesP()`函数检测线段(车道线可能是连续的线段组合):
```python
lines = cv2.HoughLinesP(binary_mask, rho=2, theta=np.pi/180, threshold=100, minLineLength=100, maxLineGap=10)
```
7. 绘制检测出的线段:
```python
for line in lines:
x1, y1, x2, y2 = line[0]
cv2.line(image, (x1, y1), (x2, y2), (0, 0, 255), 2)
```
8. 显示原始图片和检测结果:
```python
cv2.imshow("Original Image", image)
cv2.imshow("Detected Lines", binary_mask)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
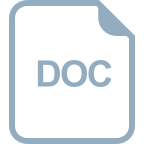
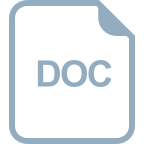
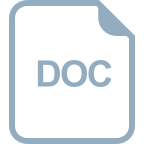
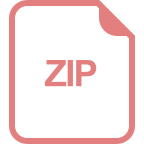
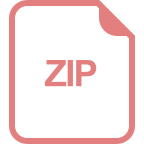
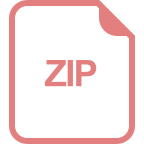
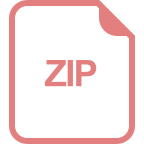
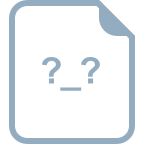
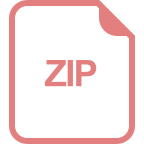
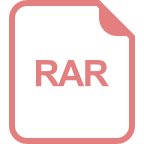
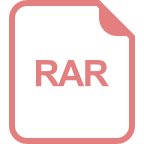
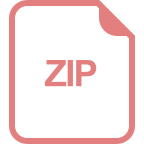
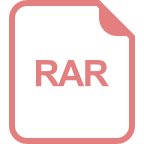
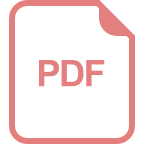
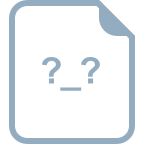
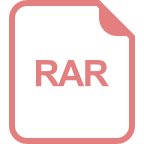
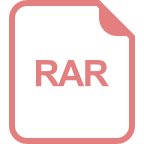