用pyqt5写一段代码:在self.label_24.show()显示的图像上鼠标点击画出红色直线,并计算直线长度
时间: 2024-12-15 07:28:45 浏览: 6
在PyQt5中,我们可以使用`QGraphicsView`和`QGraphicsScene`结合`QPainterPath`以及`QPen`来实现在图像上绘制直线并计算其长度的功能。首先,你需要创建一个`QGraphicsView`实例,然后添加一个`QGraphicsScene`并在其中加载图片。接下来,在鼠标点击事件处理器中,你可以获取点击点并绘制一条红色直线。
这是一个简单的示例代码:
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QGraphicsView, QGraphicsScene, QGraphicsLineItem, QGraphicsRectItem
from PyQt5.QtCore import Qt, QPointF
class ImageEditor(QWidget):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.view = QGraphicsView(self)
self.scene = QGraphicsScene()
self.label_24 = QLabel('Image', self)
self.image_path = 'your_image_path.jpg' # 替换为你的图片路径
self.load_image()
self.view.setScene(self.scene)
self.view.resize(800, 600)
self.view.mousePressEvent = self.on_mouse_press
def load_image(self):
pixmap = QPixmap(self.image_path)
item = QGraphicsPixmapItem(pixmap)
self.scene.addItem(item)
self.label_24.setPixmap(pixmap)
def on_mouse_press(self, event):
x = event.x()
y = event.y()
line = QGraphicsLineItem(QPointF(x, y), QPointF(event.pos().x(), event.pos().y()))
line.setPen(Qt.red) # 设置线条颜色为红色
self.scene.addItem(line)
# 计算直线长度
start_point = QPointF(x, y)
end_point = QPointF(event.pos().x(), event.pos().y())
length = (end_point - start_point).manhattanLength()
print(f"Line length: {length}")
if __name__ == "__main__":
app = QApplication(sys.argv)
widget = ImageEditor()
widget.show()
sys.exit(app.exec_())
```
在这个例子中,当你在图像上点击时,会绘制一条从点击点到新点击点的红线,并立即打印出线段的长度。记得将`your_image_path.jpg`替换为你实际需要展示的图片文件路径。
阅读全文
相关推荐
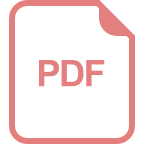
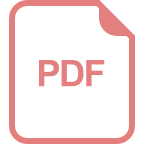
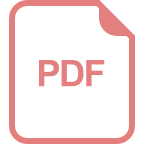
















