python体育用品销售管理系统代码
时间: 2024-07-17 08:00:48 浏览: 74
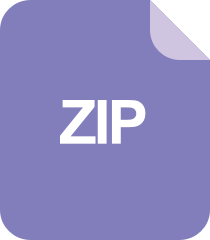
基于Python+Vue的体育用品商城管理系统设计源码
创建一个Python体育用品销售管理系统涉及到多个模块,包括用户管理、商品管理、订单处理和库存跟踪等。以下是一个简化的概念框架,而不是完整的代码:
```python
# 导入必要的库
import os
from datetime import datetime
class User:
def __init__(self, name, email, password):
self.name = name
self.email = email
self.password = password
class Product:
def __init__(self, id, name, price, stock):
self.id = id
self.name = name
self.price = price
self.stock = stock
class InventoryManager:
def __init__(self):
self.products = {} # 商品字典,id为键
def add_product(self, product):
self.products[product.id] = product
def remove_product(self, product_id):
if product_id in self.products:
del self.products[product_id]
class ShoppingCart:
def __init__(self):
self.items = {}
def add_item(self, user, product_id, quantity):
if user not in self.items:
self.items[user] = {}
self.items[user][product_id] = quantity
def remove_item(self, user, product_id):
if user in self.items and product_id in self.items[user]:
del self.items[user][product_id]
class SalesOrder:
def __init__(self, user, items, order_date):
self.user = user
self.items = items
self.order_date = order_date
def calculate_total(self):
total = sum(product.price * quantity for product_id, quantity in self.items.items())
return total
def main():
# 初始化管理器
inventory_manager = InventoryManager()
# 添加产品示例
product1 = Product(1, "Nike Running Shoes", 99.99, 10)
inventory_manager.add_product(product1)
# 用户操作示例
user = User("John Doe", "john.doe@example.com", "password")
cart = ShoppingCart()
cart.add_item(user, product1.id, 2)
# 创建订单并计算总金额
order = SalesOrder(user, cart.items, datetime.now())
total = order.calculate_total()
print(f"Order for {user.name}: Total = ${total:.2f}")
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
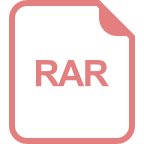
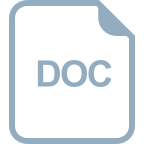
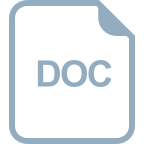
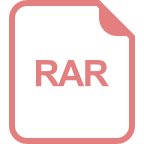
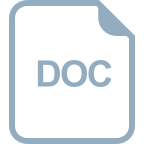
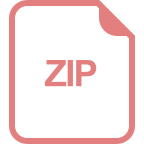
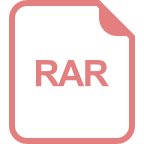
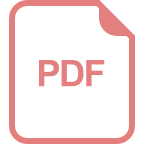
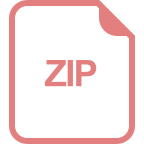
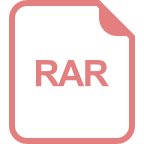
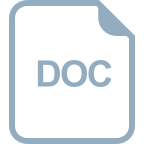
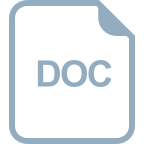
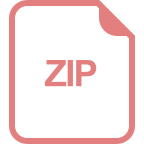
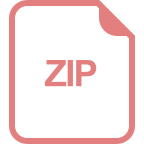
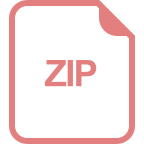
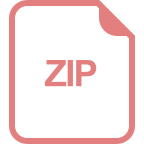
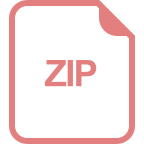