写一个go语言 DES ECB模式 ZeroPadding 填充解密的代码例子
时间: 2024-10-19 14:02:23 浏览: 15
在Go语言中,你可以使用`crypto/cipher`包提供的DES(Data Encryption Standard)加密算法,特别是ECB(Electronic Codebook)模式,它是一种简单的块密码模式,每个块独立处理。这里是一个DESECB模式下ZeroPadding填充及解密的基本示例:
```go
package main
import (
"crypto/des"
"crypto/cipher"
"fmt"
)
// 假设我们有一个明文字符串
var plaintext string = "Hello, DES!"
func main() {
// 初始化DES算法,使用默认的CBC模式,因为DESECB需要
block, err := des.NewCipher([]byte("my-secret-key")) // 使用16字节的秘钥
if err != nil {
panic(err)
}
// 创建一个DES ECB的加密上下文
ebcdEncrypter, err := cipher.NewECBEncrypter(block)
if err != nil {
panic(err)
}
// 创建一个DES ECB的解密上下文
ebcdDecrypter, err := cipher.NewECBDecrypter(block)
if err != nil {
panic(err)
}
// 拼接ZeroPadding后的原始明文
paddingSize := 8 - (len(plaintext) % 8) // 由于DES每块大小是8字节
paddedPlaintext := plaintext + string([paddingSize]byte{0})
// 分块加密
ciphertextBlocks := make([][]byte, len(paddedPlaintext)/8)
for i := 0; i < len(ciphertextBlocks); i++ {
ciphertextBlocks[i] = ecbedEncrypter.Crypt([]byte(paddedPlaintext[i*8 : (i+1)*8]), nil)
}
// 打印加密后的数据
fmt.Printf("Encrypted:\n")
for _, block := range ciphertextBlocks {
fmt.Printf("%x\n", block)
}
// 解密
plainBytes := make([]byte, len(paddedPlaintext))
for i := 0; i < len(ciphertextBlocks); i++ {
ciphertextBlocks[i] = ecbedDecrypter.Crypt(ciphertextBlocks[i], nil) // 将加密后的块解密回原始明文
copy(plainBytes[i*8 : (i+1)*8], ciphertextBlocks[i])
}
decryptedText := string(plainBytes)
// 输出解密后的文本
fmt.Printf("\nDecrypted:\n%s\n", decryptedText[:len(plaintext)]) // 我们只保留原长度的解密文本
}
```
注意:DESECB模式不适合用于保密通信,因为它不够安全,容易受到模式攻击。实际应用中应使用更现代的安全算法。
阅读全文
相关推荐
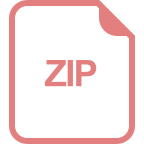
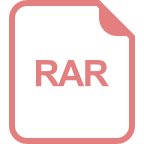

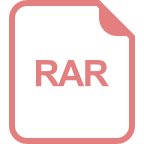
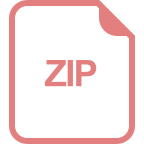
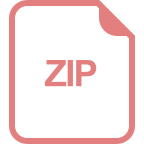
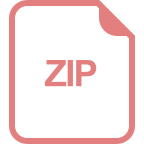
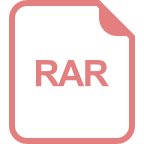
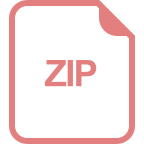
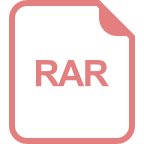
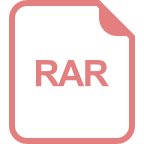
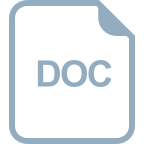




