this.tableDataList.forEach(item => { item.baTransports.forEach(items => { this.materialList.push(items) }); }); 代码优化
时间: 2024-12-19 21:22:39 浏览: 8
这段代码使用了JavaScript的箭头函数和数组方法来遍历嵌套数据结构。它首先通过`.forEach`迭代`tableDataList`中的每个元素,然后进一步迭代每个元素的`baTransports`属性。对于`baTransports`中的每一个`items`,将其添加到`materialList`中。
为了优化这段代码,我们可以考虑以下几个方面:
1. **解构赋值**:如果`items`不需要保留原始引用,可以使用解构赋值简化`push`操作,如`this.materialList.push(...items)`。
2. **避免冗余循环**:如果有条件判断需要过滤或处理某些特定情况,可以在内部循环之前添加,避免不必要的遍历。
3. **性能提升**:如果`tableDataList`非常大,可以考虑利用`Array.prototype.flat()`方法扁平化嵌套数组,然后一次性推入`materialList`。
4. **使用for-of循环**:有时候`for`循环比`forEach`更高效,尤其是在处理索引的情况下,但这里由于涉及到深度遍历,两者差异不大。
优化后的代码可能会像这样:
```javascript
if (someCondition) { // 添加条件判断
this.materialList.push(...tableDataList.flatMap(item => item.baTransports));
}
```
相关问题
优化这段代码 res.data.availablePlayItemVoList.forEach((item: any) => { this.playDateProductInfoList.forEach((v: any) => { v.playScreeningList.forEach((items: any) => { if (items.playItemCode == item.playItemCode) { this.$set( items, "availableTotal", item.availableTotal != null ? item.availableTotal : null ); } if (item.screeningList.length > 0) { item.screeningList.forEach((itemA: any) => { if (items.sysTimeQuantumList != null) { items.sysTimeQuantumList.forEach((itemB: any) => { if (itemA.screeningCode == itemB.screeningCode) { this.$set( itemB, "surplusTotal", itemA.surplusTotal != null ? itemA.surplusTotal : null ); } }); } }); } }); }); }); });
这段代码也可以使用ES6的数组方法进行优化,如下所示:
```javascript
res.data.availablePlayItemVoList.forEach(item => {
this.playDateProductInfoList.forEach(v => {
v.playScreeningList.filter(items => items.playItemCode === item.playItemCode)
.forEach(items => {
this.$set(
items,
"availableTotal",
item.availableTotal != null ? item.availableTotal : null
);
item.screeningList.forEach(itemA => {
if (items.sysTimeQuantumList != null) {
items.sysTimeQuantumList.filter(itemB => itemA.screeningCode === itemB.screeningCode)
.forEach(itemB => {
this.$set(
itemB,
"surplusTotal",
itemA.surplusTotal != null ? itemA.surplusTotal : null
);
});
}
});
});
});
});
```
同样地,使用`filter`方法可以减少循环嵌套,使代码更加清晰易读。另外,可以使用ES6的箭头函数来简化代码。
帮我优化这段代码 // res.data.availablePlayItemVoList.forEach((item: any) => { // this.playDateProductInfoList.forEach((v: any) => { // v.playScreeningList.forEach((items: any) => { // if (items.playItemCode == item.playItemCode
这段代码可以使用ES6的数组方法来进行优化,如下所示:
```javascript
res.data.availablePlayItemVoList.forEach(item => {
this.playDateProductInfoList.forEach(v => {
v.playScreeningList.filter(items => items.playItemCode === item.playItemCode)
.forEach(items => {
// your code here
});
});
});
```
使用数组的`filter`方法可以过滤出符合条件的数组元素,然后使用`forEach`方法进行遍历,这样可以减少循环嵌套的层数,使代码更加清晰易读。另外,可以使用ES6的箭头函数来简化代码。
阅读全文
相关推荐
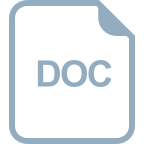
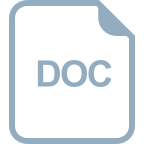
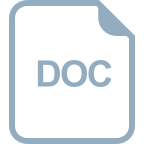
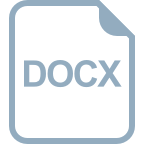
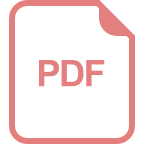
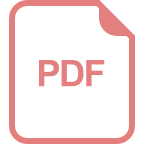
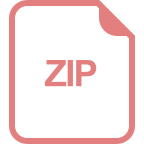
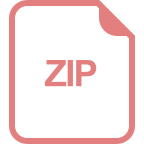
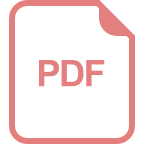
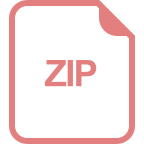
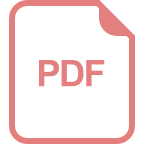
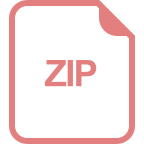
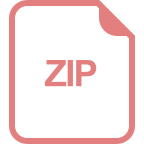
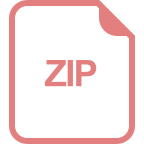
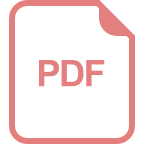