java 高效快速裁剪图片
时间: 2024-07-14 13:01:36 浏览: 105
在 Java 中高效快速地裁剪图片,可以借助第三方库,如Apache Batik 或者 ImageIO(Java自带)配合JMagick等图像处理库。以下是一个简单的示例,使用Java的ImageIO:
```java
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.Rectangle2D;
import java.io.*;
public class ImageCrop {
public static BufferedImage cropImage(String srcPath, int x, int y, int width, int height) throws IOException {
// 读取原图
BufferedImage image = ImageIO.read(new File(srcPath));
// 创建裁剪区域
Rectangle2D rect = new Rectangle2D.Float(x, y, width, height);
// 创建一个新的BufferedImage来保存裁剪结果
BufferedImage croppedImage = new BufferedImage(width, height, image.getType());
Graphics2D g2d = croppedImage.createGraphics();
g2d.setClip(rect);
g2d.drawImage(image, null, 0, 0, null);
g2d.dispose();
return croppedImage;
}
public static void main(String[] args) {
try {
String src = "path_to_your_image.jpg";
BufferedImage cropped = cropImage(src, 50, 50, 200, 200); // 裁剪位置及尺寸
saveImage(cropped, "cropped_image.jpg");
} catch (IOException e) {
e.printStackTrace();
}
}
private static void saveImage(BufferedImage img, String outputPath) throws IOException {
File output = new File(outputPath);
ImageIO.write(img, "jpg", output);
}
}
```
这个例子中,`cropImage`方法接收源路径、裁剪起始坐标和大小,然后返回一个新的已裁剪的BufferedImage。然后你可以选择将裁剪后的图像保存到文件。
相关推荐
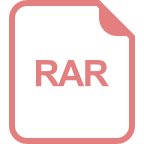
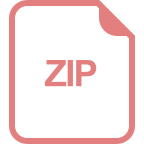
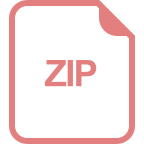














