用c++试编写一个求已知单链表的数据域的平均值的函数(数据域数据类型为整型)。
时间: 2024-11-30 19:29:15 浏览: 12
在C++中,我们可以使用递归或迭代的方式来计算单链表中所有整数元素的平均值。这里是一个简单的迭代方法示例:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val; // 数据域
ListNode* next; // 指向下一个节点的指针
};
double averageOfList(ListNode* head) {
if (head == nullptr) { // 空链表的情况
return 0.0;
}
double sum = 0;
int count = 1; // 初始化计数器
// 遍历链表
while (head != nullptr) {
sum += head->val;
head = head->next;
count++;
}
// 计算并返回平均值
return sum / count;
}
int main() {
// 创建一个测试链表
ListNode* testList = new ListNode{5};
testList->next = new ListNode{10};
testList->next->next = new ListNode{15};
double avg = averageOfList(testList);
cout << "The average of the list is: " << avg << endl;
// 释放内存
delete testList;
return 0;
}
```
这个函数`averageOfList`接受链表的头结点作为参数,通过遍历每个节点,累加所有数值,并记录节点数量,最后返回平均值。
阅读全文
相关推荐
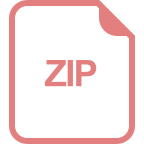
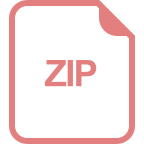
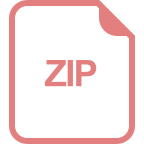
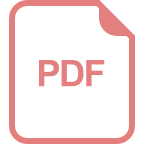
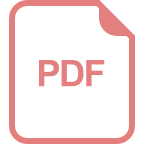
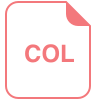
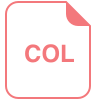
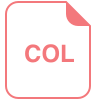
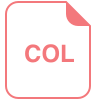
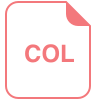
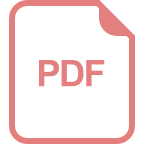
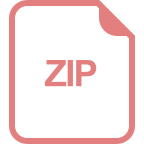
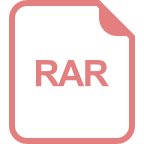
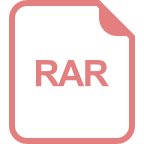
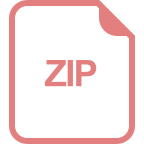
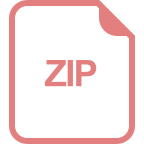