利用贪心法求解tsp问题的两种c++代码
时间: 2024-10-09 19:12:34 浏览: 74
贪心算法通常不适合精确解决旅行商问题(Traveling Salesman Problem, TSP),因为它无法保证找到全局最优解,TSP是一个已知的NP-hard问题。然而,对于一些启发式近似算法,比如"Nearest Neighbor"(最近邻)和"Two-Opt"(两交换),可以利用贪心策略来简化问题。
以下是两个简单的示例,它们不是完整的解决方案,而是展示了如何使用贪心策略在C++中处理TSP:
1. Nearest Neighbor (NN)算法:
```cpp
#include <vector>
#include <algorithm>
struct Point {
int x, y;
};
bool compareDist(const Point& a, const Point& b) {
return std::hypot(a.x - b.x, a.y - b.y) < std->hypot(b.x - a.x, b.y - a.y);
}
std::vector<Point> nearestNeighbor(std::vector<Point>& cities) {
std::sort(cities.begin(), cities.end(), compareDist);
std::vector<int> tour;
tour.push_back(0);
for (size_t i = 1; i < cities.size(); ++i) {
tour.push_back(i);
}
// 添加回起点
tour.push_back(0);
return tour;
}
```
这个版本只保证了每次选择离当前路径最近的城市,结果不一定是最优的。
2. Two-Opt 算法(仅用于改进已有的路径):
```cpp
void twoOptImprovement(std::vector<int>& tour, std::vector<Point>& cities) {
for (int i = 1; i < tour.size() - 1; i++) {
for (int j = i + 2; j < tour.size(); j++) {
std::vector<int> temp(tour.begin(), tour.begin() + i);
temp.insert(temp.end(), tour.begin() + j, tour.end());
temp.pop_back();
// 计算新路径长度并比较
if (calcDistance(cities, temp) < calcDistance(cities, tour)) {
tour = temp;
}
}
}
}
// 假设calcDistance函数计算两点之间的曼哈顿距离或其他合适的距离
```
这展示了如何通过改变路径顺序(两交换操作)来尝试优化已存在的路径,但同样不保证得到全局最优解。
阅读全文
相关推荐
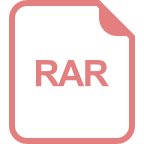
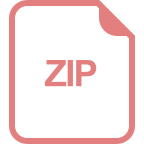
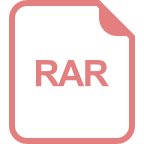

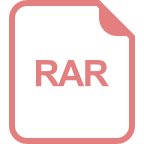
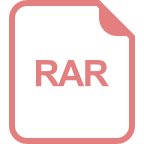
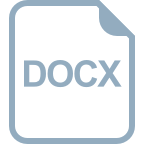
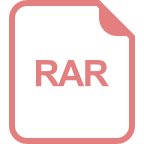
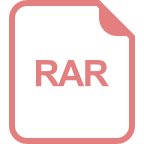
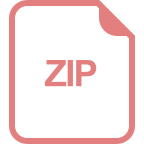
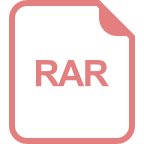
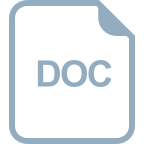
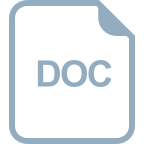
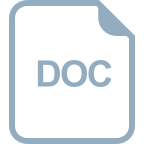
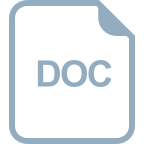
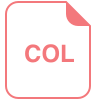
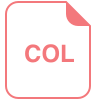
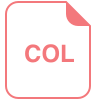