根据所学线性表的类型定义等相关知识,完成顺序表算法库的建立。
时间: 2024-09-26 12:18:19 浏览: 40
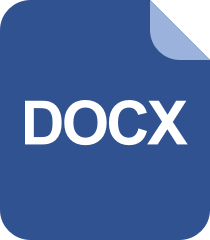
数据结构学习--线性表及其应用--顺序表

顺序表是一种基于数组的数据结构,它按照元素在内存中的存储顺序来组织数据。为了创建一个顺序表的算法库,我们可以实现以下几个核心操作:
1. **初始化** (CreateList): 创建一个空的顺序表,给定表的大小。
```c++
struct Node {
int data;
Node* next; // 指向下一个节点的指针
};
SequenceList* CreateList(int capacity) {
SequenceList* list = new SequenceList();
list->size = 0;
list->capacity = capacity;
list->head = NULL;
return list;
}
```
2. **插入** (Insert): 在指定位置插入新元素。如果需要动态扩容,需要处理边界情况。
```c++
void Insert(SequenceList* list, int index, int value) {
if (index < 0 || index > list->size) {
printf("Error: Index out of bounds.\n");
return;
}
if (list->size == list->capacity) {
ResizeList(list);
}
Node* newNode = new Node{value, list->head};
for (int i = 0; i < index - 1; ++i) {
list->data[i] = list->data[i + 1];
}
list->data[index] = newNode->data;
list->size++;
}
```
3. **删除** (Delete): 删除指定索引处的元素。
```c++
void Delete(SequenceList* list, int index) {
if (index < 0 || index >= list->size) {
printf("Error: Index out of bounds.\n");
return;
}
Node* temp = list->head;
for (int i = 0; i < index; ++i) {
temp = temp->next;
}
delete temp->next;
list->data[index] = temp->data;
list->size--;
if (index == list->size && list->size == list->capacity / 4) {
ShrinkList(list);
}
}
```
4. **搜索** (Search): 查找指定值的元素。
```c++
bool Search(SequenceList* list, int value) {
Node* current = list->head;
while (current != NULL) {
if (current->data == value) {
return true;
}
current = current->next;
}
return false;
}
```
5. **其他实用函数** (例如:获取长度、访问元素等)
注意:`ResizeList()` 和 `ShrinkList()` 函数用于调整列表容量以提高效率。
阅读全文
相关推荐
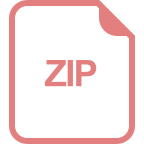
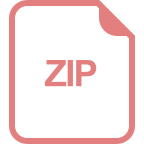
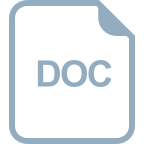
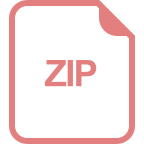
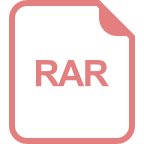
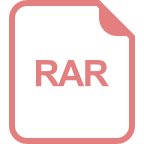
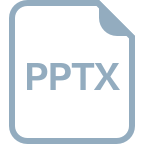
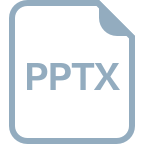
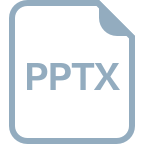
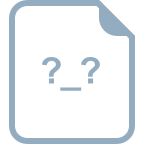
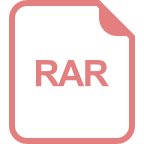
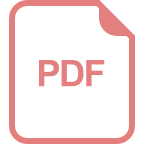
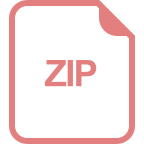