1.1 设置一个灯每秒闪烁一次; 1.2设计流水灯,每秒移动一位; 1.3设计一个开关,通过查询方式读入开关状态,控制流水灯移动的方向。
时间: 2024-09-30 16:15:00 浏览: 79
在编程中,设置一个灯每秒闪烁一次通常涉及定时任务。这里是一个简单的Python示例,假设我们有LED灯对象`light`:
```python
import time
# 定义灯类
class Light:
def flash(self):
print("灯闪烁!")
# 模拟灯闪操作
time.sleep(1) # 等待1秒
# 实例化灯并设置每秒闪烁
lamp = Light()
while True:
lamp.flash()
time.sleep(1)
```
对于设计流水灯,可以将灯看作数组,每秒改变一位的位置。同样用Python编写:
```python
class WaterfallLight:
def __init__(self, lights=5):
self.lights = list(range(lights))
def move(self):
if len(self.lights) > 0:
self.lights.append(0) # 新位置添加到末尾
self.lights.pop(0) # 移除当前位置
def show(self):
print("流水灯当前位置:", self.lights)
waterfall = WaterfallLight()
while True:
waterfall.move()
waterfall.show()
time.sleep(1)
```
对于开关控制,我们可以创建一个Switch类,通过查询属性来获取开关状态,并更新灯的移动方向:
```python
class Switch:
def __init__(self):
self.status = "开"
def query_status(self):
return self.status
def toggle_direction(self):
self.status = "关" if self.status == "开" else "开"
print(f"开关状态已更改为:{self.status}")
switch = Switch()
while True:
direction = switch.query_status()
if direction == "开":
waterfall.move_right() # 假设move_right()用于向右移动
else:
waterfall.move_left() # move_left()用于向左移动
time.sleep(1)
```
阅读全文
相关推荐
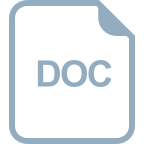
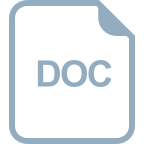
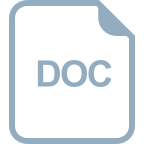
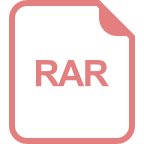
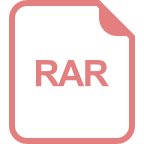
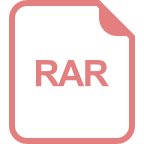
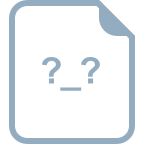
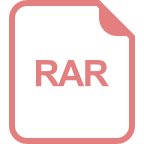
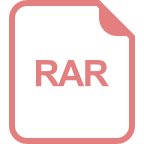
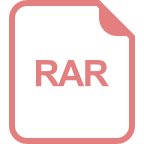
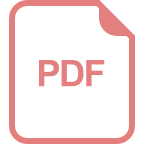
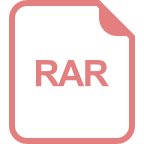
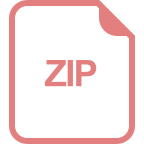
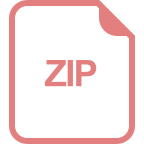
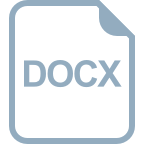
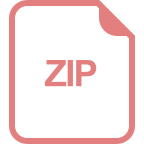
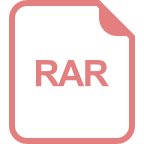
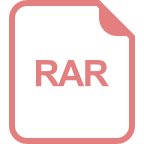