自定义学生类:包含姓名,年龄,成绩 属性为私有成员变量,并生成无参,有参构造方法,生成get/set方法.创建5个学生放到 ArrayList中.使用迭代器获取每个学生信息.统计总分,平均分,最高分,最低分并输出。(实现思路如下:) ⦁ 定义学生类生成类无参,有参构造方法,生成get/set方法. ⦁ 定义ArrayList ⦁ 添加5个学生 ⦁ 获取迭代器 ⦁ 定义总分 ⦁ 定义最高分 ⦁ 定义最低分 ⦁ 循环判断是否有下一个学生 ⦁ 使用next方法获取学生对象 ⦁ 将这个学生对象的分数添加到总分 ⦁ 判断这个学生的分数是否大于最大分数 ⦁ 这个学生的分数大于最大分数.最大分数等于这个学生的分数 ⦁ 判断这个学生的分数是否大低最大分数 ⦁ 这个学生的分数小于最小分数.最小分数等于这个学生的分数 ⦁ 计算平均分(平均分 = 总分/人数) ⦁ 打印总分,最高分,最低分
时间: 2024-04-15 12:31:18 浏览: 19
以下是一个示例的自定义学生类的实现代码:
```java
import java.util.ArrayList;
import java.util.Iterator;
public class Student {
private String name;
private int age;
private int score;
public Student() {}
public Student(String name, int age, int score) {
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public int getScore() {
return score;
}
public void setScore(int score) {
this.score = score;
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("Alice", 18, 90));
students.add(new Student("Bob", 19, 85));
students.add(new Student("Charlie", 20, 95));
students.add(new Student("David", 21, 88));
students.add(new Student("Eve", 22, 92));
Iterator<Student> iterator = students.iterator();
int totalScore = 0;
int highestScore = Integer.MIN_VALUE;
int lowestScore = Integer.MAX_VALUE;
while (iterator.hasNext()) {
Student student = iterator.next();
totalScore += student.getScore();
highestScore = Math.max(highestScore, student.getScore());
lowestScore = Math.min(lowestScore, student.getScore());
}
int averageScore = totalScore / students.size();
System.out.println("总分:" + totalScore);
System.out.println("平均分:" + averageScore);
System.out.println("最高分:" + highestScore);
System.out.println("最低分:" + lowestScore);
}
}
```
以上代码中,我们首先定义了一个学生类 `Student`,包含私有的姓名、年龄和成绩属性,以及对应的构造方法和 getter/setter 方法。
然后,在 `Main` 类中,我们创建了一个 `ArrayList` 来存储学生对象,并添加了5个学生。然后使用迭代器来遍历每个学生对象,计算总分、最高分和最低分。最后,计算平均分并输出结果。
注意,这里的平均分是使用整数除法计算的,如果需要保留小数位数,可以将 `totalScore` 和 `students.size()` 都转换为浮点数进行计算。
相关推荐
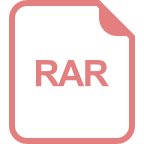
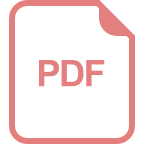
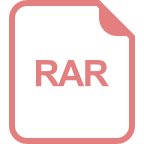












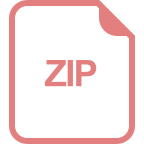
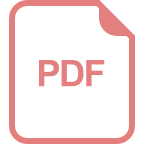
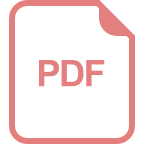
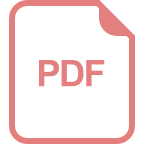