添加功能:1.标题栏再加上下划线;2.鼠标移动到按钮上时,对应的按钮会高亮
时间: 2024-04-16 22:28:32 浏览: 160
要实现标题栏的下划线和按钮高亮的功能,你可以使用 `javax.swing.border` 包中的 `MatteBorder` 类来创建下划线效果,并使用鼠标事件监听器来实现按钮的高亮效果。以下是修正后的代码:
```java
import javax.swing.*;
import javax.swing.border.LineBorder;
import javax.swing.border.MatteBorder;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
public class CustomWindow extends JFrame {
private JPanel titleBar;
private JButton minimizeButton;
private JButton maximizeButton;
private JButton closeButton;
public CustomWindow() {
setSize(400, 300);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setUndecorated(true); // 去掉窗口边框默认样式
// 创建标题栏
createTitleBar();
getContentPane().setBackground(Color.WHITE); // 设置窗口背景色为白色
setVisible(true);
}
private void createTitleBar() {
// 创建标题栏面板
titleBar = new JPanel();
titleBar.setBackground(Color.WHITE);
titleBar.setPreferredSize(new Dimension(getWidth(), 30));
// 添加最小化按钮
minimizeButton = createTitleBarButton("-");
minimizeButton.addActionListener(e -> setState(JFrame.ICONIFIED));
// 添加最大化/还原按钮
maximizeButton = createTitleBarButton("□");
maximizeButton.addActionListener(e -> {
if (getExtendedState() == JFrame.MAXIMIZED_BOTH) {
setExtendedState(JFrame.NORMAL);
} else {
setExtendedState(JFrame.MAXIMIZED_BOTH);
}
});
// 添加关闭按钮
closeButton = createTitleBarButton("✕");
closeButton.addActionListener(e -> {
dispose();
System.exit(0);
});
// 添加按钮到标题栏
titleBar.setLayout(new FlowLayout(FlowLayout.RIGHT));
titleBar.add(minimizeButton);
titleBar.add(maximizeButton);
titleBar.add(closeButton);
// 添加鼠标监听器以实现拖动窗口功能和按钮高亮效果
titleBar.addMouseListener(new MouseAdapter() {
public void mousePressed(MouseEvent e) {
initialClick = e.getPoint();
getComponentAt(initialClick);
}
public void mouseEntered(MouseEvent e) {
setButtonHighlight(true);
}
public void mouseExited(MouseEvent e) {
setButtonHighlight(false);
}
});
titleBar.addMouseMotionListener(new MouseAdapter() {
public void mouseDragged(MouseEvent e) {
int thisX = getLocation().x;
int thisY = getLocation().y;
int xMoved = e.getX() - initialClick.x;
int yMoved = e.getY() - initialClick.y;
int x = thisX + xMoved;
int y = thisY + yMoved;
setLocation(x, y);
}
});
// 将标题栏面板添加到窗口的顶部
getContentPane().add(titleBar, BorderLayout.NORTH);
}
private void setButtonHighlight(boolean isHighlighted) {
Color highlightColor = new Color(230, 230, 230); // 高亮颜色
if (isHighlighted) {
minimizeButton.setBackground(highlightColor);
maximizeButton.setBackground(highlightColor);
closeButton.setBackground(highlightColor);
} else {
minimizeButton.setBackground(Color.WHITE);
maximizeButton.setBackground(Color.WHITE);
closeButton.setBackground(Color.WHITE);
}
}
private JButton createTitleBarButton(String text) {
JButton button = new JButton(text);
button.setFocusPainted(false);
button.setBackground(Color.WHITE);
button.setBorder(null);
button.setPreferredSize(new Dimension(30, 30));
return button;
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
CustomWindow window = new CustomWindow();
});
}
}
```
在这个修正后的代码中,我们添加了 `setButtonHighlight()` 方法来设置按钮的高亮效果。当鼠标进入标题栏时,我们调用该方法将按钮的背景色设置为高亮颜色;当鼠标离开标题栏时,将按钮的背景色恢复为白色。
另外,我们使用了 `MatteBorder` 类创建了标题栏的下划线效果。在 `createTitleBar()` 方法中,你可以添加以下代码来创建下划线:
```java
// 创建下划线边框
MatteBorder underlineBorder = new MatteBorder(0, 0, 1, 0, Color.WHITE);
titleBar.setBorder(underlineBorder);
```
希望这个修正后的代码能满足你的需求!
阅读全文
相关推荐
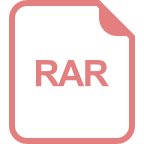







