if vg.name not in bones_to_delete: UnicodeDecodeError: 'utf-8' codec can't decode byte 0xf8 in position 0: invalid start byte
时间: 2024-06-05 15:04:14 浏览: 170
这是一个Unicode解码错误,通常是因为字符串中包含了无法被解码的字节,而utf-8编码无法解码这些字节。解决该问题的方法取决于具体情况,但以下是一些可能的解决方法:
1. 检查字符串是否采用了正确的编码方式,比如是否应该使用gbk编码而不是utf-8编码。
2. 尝试使用Python内置的错误处理方法,如在打开文件时使用`open(filename, encoding="utf-8", errors="ignore")`来忽略无法解码的字符。
3. 如果无法确定文件的编码方式,可以尝试使用第三方库chardet来自动检测文件的编码方式。
相关问题
if vg.name not in bones_to_delete: UnicodeDecodeError: 'utf-8' codec can't decode byte 0xf8 in position 0: invalid start byte
这个错误通常是由于编码问题引起的。当Python尝试使用utf-8编码解码包含非utf-8字符的字符串时,就会出现这个错误。
在这个特定的错误中,可能是因为vg.name包含了无法使用utf-8编码的字符,导致Python无法解码该字符串。
你可以尝试使用其他编码方式来解决这个问题,或者检查vg.name是否包含非法字符。另外,也可以尝试使用try except语句来处理这个错误,以便程序可以继续运行。
Write java code: Copy the files, small_weapons.txt, and large_weapons.txt from the assignment folder on Blackboard and save them to your folder. For testing purposes, you should use the small file. Use the large file when you think the application works correctly. To see what is in the files use a text editor. Nilesh is currently enjoying the action RPG game Torchlight 2 which is an awesome game and totally blows Auction House Simulator 3, oh sorry, that should be Diablo 3, out of the water. He has got a file containing info on some of the unique weapons in the game. The transaction file contains the following information: Weapon Name (string) Weapon Type (string) Damage (int) Weapon Speed (double) … To tell if one weapon is better than another you need to know the Damage Per Second (DPS) the weapon does, since weapons have a different attack speed. DPS is calculated by taking the damage value and dividing it by the attack speed.a) You will write a program that will allow Nilesh to load the file and display the weapon info and DPS of every weapon in the input file. When the user chooses to open a weapon file, they are required to type in the filename. It will then read the 4 values about a particular weapon and then display the 4 values to the console window on one line neatly padded along with the DPS of the weapon. This is repeated until the end of the file. b) Modify your code from a) so that the weapon information written to the console window is also written to a text file. The user should be able to specify the name of the file. Add a header to the beginning of the file which has column headers for each column. At the end of the text file display the total number of weapons in the file. c) Document your code appropriately and add your name and id number as comments at the top of your code. Please also submit this text file you have created. Enter the file you want to search end with .txt: large_weapons.txt 1 Blackfang Bludgeon Great Hammer 489 1.44 339.58333333333337 2 Bones 2 Boneshredder Great Axe 256 0.84 304.76190476190476 3 Comet's Tail Great Sword 872 1.2 726.6666666666667 4 Decapitator Great Sword 188 1.08 174.07407407407408 5 Demolisher Great Hammer 887 1.32 671.9696969696969
```
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class WeaponInfo {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the file you want to search end with .txt: ");
String fileName = scanner.nextLine();
File file = new File(fileName);
try {
Scanner fileScanner = new Scanner(file);
FileWriter writer = new FileWriter(new File("weapon_info_output.txt"));
writer.write("Weapon Name\tWeapon Type\tDamage\tWeapon Speed\tDPS\n");
int count = 0;
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
String[] tokens = line.split(" ");
String weaponName = tokens[0];
String weaponType = tokens[1];
int damage = Integer.parseInt(tokens[2]);
double weaponSpeed = Double.parseDouble(tokens[3]);
double dps = damage / weaponSpeed;
System.out.printf("%-20s%-20s%-10d%-15.2f%.2f\n", weaponName, weaponType, damage, weaponSpeed, dps);
writer.write(weaponName + "\t" + weaponType + "\t" + damage + "\t" + weaponSpeed + "\t" + dps + "\n");
count++;
}
System.out.println("Total number of weapons: " + count);
writer.write("Total number of weapons: " + count);
writer.close();
fileScanner.close();
} catch (IOException e) {
System.out.println("Unable to read file: " + fileName);
} catch (NumberFormatException e) {
System.out.println("Invalid file format: " + fileName);
}
scanner.close();
}
}
```
阅读全文
相关推荐
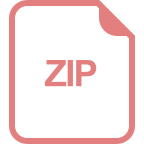
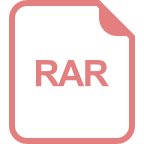
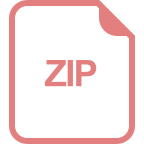
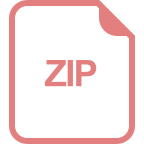
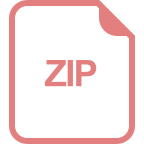
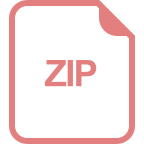
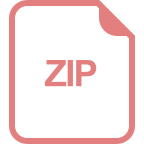
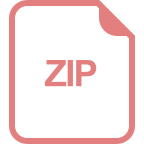
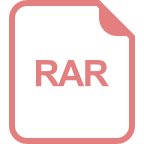
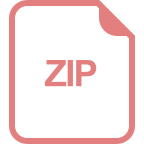
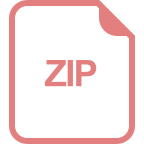
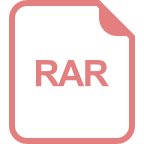
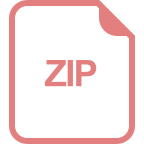
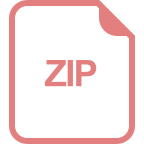
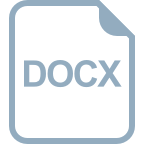
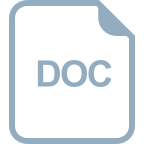