word2vec-bilstm-crf
时间: 2023-12-18 18:04:42 浏览: 163
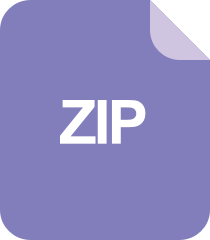
word2vec
Word2vec-BiLSTM-CRF是一种用于命名实体识别(NER)的神经网络模型。它结合了三个不同的层:Word2vec嵌入层、双向长短时记忆网络(BiLSTM)层和条件随机场(CRF)层。Word2vec嵌入层将每个单词转换为向量表示,BiLSTM层将这些向量作为输入并学习上下文信息,最后CRF层将BiLSTM层的输出作为输入并进行标记预测。
以下是Word2vec-BiLSTM-CRF模型的基本步骤:
1. 将每个单词转换为向量表示,这可以使用预训练的Word2vec模型来完成。
2. 将这些向量作为输入传递给BiLSTM层,该层将学习上下文信息并生成相应的输出。
3. 将BiLSTM层的输出作为输入传递给CRF层,该层将对每个标记进行预测,并使用Viterbi算法来找到最佳标记序列。
以下是一个使用Python和Keras实现Word2vec-BiLSTM-CRF模型的示例:
```python
from keras.models import Model, Input
from keras.layers import LSTM, Embedding, Dense, TimeDistributed, Dropout, Bidirectional
from keras_contrib.layers import CRF
import numpy as np
# 定义模型输入
input = Input(shape=(MAX_LEN,))
# 添加Word2vec嵌入层
model = Embedding(input_dim=len(word2idx), output_dim=EMBEDDING_DIM, input_length=MAX_LEN, weights=[embedding_matrix], trainable=False)(input)
# 添加双向LSTM层
model = Bidirectional(LSTM(units=HIDDEN_UNITS, return_sequences=True, recurrent_dropout=0.1))(model)
# 添加Dropout层
model = Dropout(0.1)(model)
# 添加全连接层
model = TimeDistributed(Dense(units=NUM_TAGS, activation="relu"))(model)
# 添加CRF层
crf = CRF(NUM_TAGS)
out = crf(model)
# 定义模型
model = Model(input, out)
# 编译模型
model.compile(optimizer="rmsprop", loss=crf.loss_function, metrics=[crf.accuracy])
# 训练模型
model.fit(X_train, np.array(y_train), batch_size=BATCH_SIZE, epochs=EPOCHS, validation_split=0.1, verbose=1)
# 预测标记
y_pred = model.predict(X_test)
```
阅读全文
相关推荐
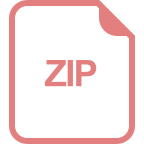
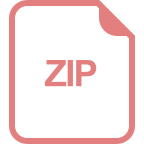

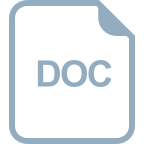


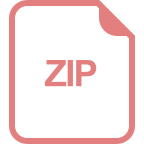
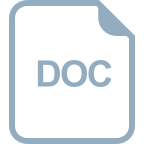
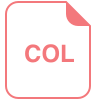
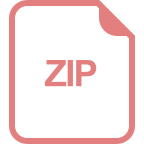
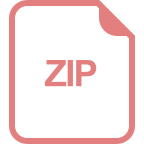
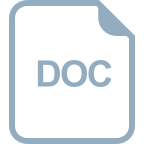
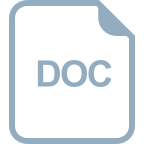
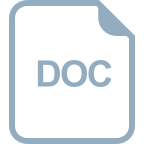
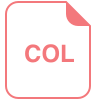


