单链表操作及实现包括查找插入删除数据
时间: 2024-09-24 22:03:36 浏览: 8
在单链表中,常见的操作主要包括查找、插入和删除数据。以下是这三种操作的基本实现:
1. 查找(Search):
```c
Node* search(Node* head, int value) {
Node* current = head;
while (current != NULL) {
if (current->data == value) {
return current; // 找到了就直接返回
}
current = current->next;
}
return NULL; // 如果未找到,返回NULL
}
```
2. 插入(Insertion):
```c
void insertAtPosition(Node** head, int value, int position) {
// 具体参照上文创建节点和插入节点的函数
}
```
3. 删除(Deletion):
```c
void deleteNode(Node** head, int value) {
if (*head == NULL) {
return;
}
if ((*head)->data == value) {
Node* temp = *head;
*head = (*head)->next;
free(temp); // 释放已删除节点的内存
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
Node* toDelete = current->next;
current->next = current->next->next;
free(toDelete); // 释放已删除节点的内存
} else {
printf("Element not found.\n");
}
}
```
在`main()`函数中,你可以结合上述函数使用,比如先查找元素,然后插入或删除。记得处理特殊情况,如空链表或目标元素不存在等情况。
相关推荐
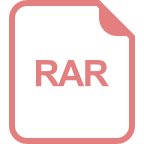
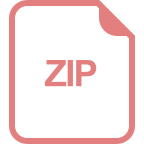
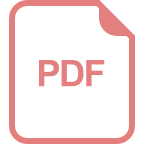














