用字符数组完成:输入10个字符,统计其中大写,小写,数字字符的个数。
时间: 2024-11-20 14:40:48 浏览: 5
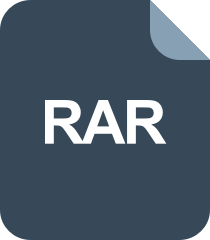
tj.rar_visual c_数字字符个数
要使用字符数组完成这个功能,你需要首先接收用户的输入,然后遍历这个数组以判断每个字符是大写字母、小写字母还是数字。这里是一个简单的C语言程序示例:
```c
#include <stdio.h>
int is_upper(char c) {
return (c >= 'A' && c <= 'Z');
}
int is_lower(char c) {
return (c >= 'a' && c <= 'z');
}
int is_digit(char c) {
return (c >= '0' && c <= '9');
}
void count_chars(char arr[], int len) {
int upper_count = 0, lower_count = 0, digit_count = 0;
for (int i = 0; i < len; i++) {
if (is_upper(arr[i])) {
upper_count++;
} else if (is_lower(arr[i])) {
lower_count++;
} else if (is_digit(arr[i])) {
digit_count++;
}
}
printf("Uppercase characters: %d\n", upper_count);
printf("Lowercase characters: %d\n", lower_count);
printf("Digit characters: %d\n", digit_count);
}
int main() {
char input[11]; // Account for null terminator
printf("Enter 10 characters: ");
fgets(input, sizeof(input), stdin); // Read user input with newline
// Remove the newline character from input
size_t newline_index = strlen(input) - 1;
if (input[newline_index] == '\n') {
input[newline_index] = '\0';
}
count_chars(input, strlen(input));
return 0;
}
```
在这个程序中,我们定义了三个辅助函数 `is_upper`、`is_lower` 和 `is_digit` 来检查字符是否为大写字母、小写字母或数字。`count_chars` 函数遍历输入数组并统计相应的字符数量。`main` 函数则获取用户输入并调用 `count_chars`。
阅读全文
相关推荐
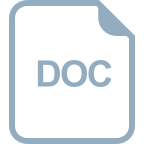
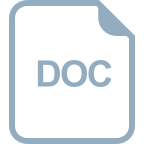















