python绘制圆形
时间: 2024-09-20 16:10:22 浏览: 42
在Python中,你可以使用matplotlib库来绘制圆形,这是一个非常常用的数据可视化工具。以下是使用`plt.Circle`函数创建简单圆形的基本步骤:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一个新的图形窗口
fig, ax = plt.subplots()
# 定义圆心位置和半径
center = (0.5, 0.5)
radius = 0.3
# 创建一个圆形 patches
circle = plt.Circle(center, radius, fill=False) # 如果你想填充圆形,可以将fill设为True
# 将圆形添加到当前的坐标轴上
ax.add_patch(circle)
# 设置坐标轴范围,包括圆心
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
# 显示图形
plt.show()
```
在这个例子中,`plt.Circle`返回的是一个圆形对象,然后通过`ax.add_patch()`将其添加到绘图区域。如果你想让圆形有颜色,可以在创建Circle时指定`color`参数。
相关问题
Python绘制圆形螺旋
Python中可以使用turtle库绘制圆形螺旋,具体实现如下:
```python
import turtle
t = turtle.Turtle()
t.speed(0)
for i in range(1000):
t.circle(5*i)
t.circle(-5*i)
t.left(i)
```
以上代码中,首先导入了turtle库,并创建了一个Turtle对象。然后将画笔速度设置为0,即最快速度。接着使用for循环绘制圆形螺旋,每次循环都通过调用circle()方法绘制一个半径为5i的圆,然后绘制一个半径为-5i的圆,这样就会形成一个螺旋的效果。最后每次循环将画笔左转i度。
python 绘制椭圆形mask
你可以使用 Python 中的 PIL(Python Imaging Library)模块来绘制椭圆形的 mask,具体步骤如下:
1. 导入 PIL 模块:
```python
from PIL import Image, ImageDraw
```
2. 创建一个空白的图像并获取其 Draw 对象:
```python
img = Image.new('L', (width, height), 0)
draw = ImageDraw.Draw(img)
```
其中,'L' 表示图像的模式为灰度图像,(width, height) 表示图像的大小,0 表示图像的初始颜色为黑色。
3. 绘制椭圆形:
```python
draw.ellipse((x0, y0, x1, y1), fill=255)
```
其中,(x0, y0) 和 (x1, y1) 分别表示椭圆形的左上角和右下角的坐标,fill=255 表示椭圆形的颜色为白色。
4. 获取椭圆形的 mask:
```python
mask = img.convert('1')
```
其中,'1' 表示将图像转换为二值图像(即黑白图像),mask 中的白色像素即为椭圆形所覆盖的像素,黑色像素则表示未被覆盖的像素。
完整的代码示例:
```python
from PIL import Image, ImageDraw
# 创建一个空白的图像并获取其 Draw 对象
width, height = 512, 512
img = Image.new('L', (width, height), 0)
draw = ImageDraw.Draw(img)
# 绘制椭圆形
x0, y0, x1, y1 = 100, 100, 400, 300
draw.ellipse((x0, y0, x1, y1), fill=255)
# 获取椭圆形的 mask
mask = img.convert('1')
# 显示图像和 mask
img.show()
mask.show()
```
阅读全文
相关推荐
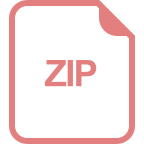
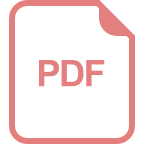
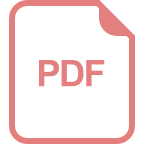













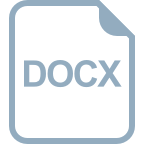