C++ struct 运算符重载
时间: 2024-03-18 09:34:53 浏览: 185
C++中的struct可以通过运算符重载来定义自己的语义。运算符重载允许用户为该运算符指定自定义语义,当它们与用户定义的类型一起使用时,可以更加方便地进行操作。在struct中,运算符重载可以通过成员函数或者非成员函数来实现。成员函数的格式为operator运算符(),而非成员函数的格式为operator运算符(参数列表)。其中,参数列表中至少有一个参数是struct类型。在运算符重载中,我们可以自定义运算符的行为,例如比较大小、相加等等。需要注意的是,运算符重载不会改变运算符的优先级和结合性。
相关问题
struct重载运算符
### C++ 结构体重载运算符示例与规则
在C++中,可以在结构体(`struct`)内部定义成员函数来实现运算符重载。这使得可以自定义特定类型的对象之间的操作行为。
#### 运算符重载的一般形式
对于二元运算符,通常的形式如下:
```cpp
struct MyStruct {
// 成员变量...
// 重载二元运算符
MyStruct operator+(const MyStruct& other) const;
};
```
而对于一元运算符,则不需要参数传递另一个实例:
```cpp
struct MyStruct {
// 成员变量...
// 重载一元运算符
MyStruct operator-() const;
};
```
#### 实际例子:整数向量类中的加法运算符重载
下面是一个具体的例子,在这个例子中创建了一个简单的二维向量类,并实现了两个向量相加的功能[^1]:
```cpp
#include <iostream>
struct Vector2D {
double x, y;
// 构造函数初始化列表用于设置默认值或接收外部传入的坐标值
Vector2D(double _x = 0.0, double _y = 0.0): x(_x), y(_y){}
// 定义加法运算符的行为
Vector2D operator+(const Vector2D &other) const {
return Vector2D(x + other.x, y + other.y);
}
friend std::ostream& operator<<(std::ostream &os, const Vector2D &v){
os << "(" << v.x << ", " << v.y << ")";
return os;
}
};
int main(){
Vector2D vecA(3.5, 7.8);
Vector2D vecB(-2.4, 6.9);
Vector2D resultVec = vecA + vecB;
std::cout << "Resultant vector is: " << resultVec << '\n';
return 0;
}
```
此代码片段展示了如何在一个名为 `Vector2D` 的简单结构体内重载加号(`+`)运算符以支持两维向量间的加法计算。此外还提供了一种友元函数的方式重载流插入运算符 (`<<`) 来方便打印输出结果。
#### 关于赋值运算符和其他特殊运算符
当涉及到像复制构造函数、移动语义以及析构函数这样的特殊情况时,应该特别小心处理资源管理问题。如果结构体包含指针或其他动态分配的数据成员,则可能还需要显式地定义这些特殊的成员函数来确保正确性和效率。
c++不用运算符重载进行复数加减法
在 C++ 中,可以使用结构体或类来表示复数,然后定义成员函数实现加减法运算。以下是一个示例代码:
```c++
#include <iostream>
using namespace std;
struct Complex {
double real, imag;
Complex(double r = 0.0, double i = 0.0): real(r), imag(i) {}
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
void display() const {
cout << "(" << real << ", " << imag << "i)" << endl;
}
};
int main() {
Complex c1(1.0, 2.0);
Complex c2(3.0, 4.0);
Complex c3 = c1 + c2;
Complex c4 = c1 - c2;
c3.display();
c4.display();
return 0;
}
```
在上面的代码中,定义了一个结构体 Complex,其中包含实部和虚部两个成员变量,以及加减法运算符重载的成员函数。在主函数中,创建了两个复数对象 c1 和 c2,并分别进行加减法运算,将结果保存到 c3 和 c4 中,并输出结果。
阅读全文
相关推荐
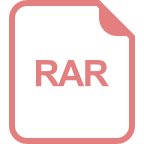















