基于java的gui中按钮怎么显示输入的内容
时间: 2024-11-03 20:19:06 浏览: 18
在Java GUI中,如果你想要创建一个按钮,当用户点击它时显示他们输入的内容,通常可以这样做:
1. **JButton和ActionListener**:
使用`javax.swing.JButton`类创建一个按钮,并通过`addActionListener()`方法添加一个监听器。在这个监听器中,你可以定义一个`ActionEvent`处理函数,在用户点击按钮时执行。
```java
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main {
private JTextField textField; // 输入框
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
JFrame frame = new JFrame("Input Button");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new FlowLayout());
textField = new JTextField(10); // 创建文本字段
JButton button = new JButton("Display Input"); // 创建按钮
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String userInput = textField.getText(); // 获取输入内容
JOptionPane.showMessageDialog(frame, "User Input: " + userInput); // 显示在消息框中
}
});
frame.add(textField);
frame.add(button);
frame.pack();
frame.setVisible(true);
});
}
}
```
2. **SwingWorker** 或者 **Background Thread**:
如果你想在后台处理用户的输入并更新UI,可以使用`SwingWorker`或在一个单独的线程中操作。这样可以保持GUI响应。
```java
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.concurrent.Callable;
class Worker extends SwingWorker<String, Void> {
@Override
protected String doInBackground() throws Exception {
while (!isCancelled()) { // 模拟等待用户输入
textField.setText("Waiting for input...");
try {
Thread.sleep(500);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
return textField.getText();
}
@Override
protected void done() {
if (!isCancelled()) {
try {
String userInput = get();
SwingUtilities.invokeLater(() -> JOptionPane.showMessageDialog(frame, "User Input: " + userInput));
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
public class Main {
// ...
public static void main(String[] args) {
// ...
JButton button = new JButton("Display Input");
button.addActionListener(e -> new Worker().execute()); // 添加Worker到按钮的事件监听
// ...
}
}
```
阅读全文
相关推荐





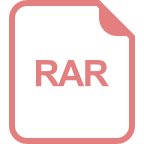












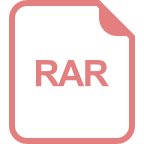