不通过调用别的库,C++求点集最小包围球保证结果唯一性的详细代码
时间: 2024-12-10 10:54:42 浏览: 5
在C++中,计算点集的最小包围球通常涉及到找到所有点到某一点距离的平方和最小的一组点。由于直接找出所有点对之间的最短距离可能会非常耗时,我们可以采用一种近似算法,比如Welzl's Algorithm,它可以在O(n)的时间复杂度内找到近似的最优解。这里提供一个基本的思路:
```cpp
#include <vector>
#include <cmath>
// 定义三维向量结构体
struct Point {
double x, y, z;
};
// 计算两个点的距离平方
double distance_squared(const Point& p1, const Point& p2) {
return (p1.x - p2.x) * (p1.x - p2.x) + (p1.y - p2.y) * (p1.y - p2.y) + (p1.z - p2.z) * (p1.z - p2.z);
}
// Welzl's Algorithm求最小包围球
Point min_enclosing_sphere(std::vector<Point>& points) {
// 初始化一个随机点作为初始中心
Point center = points[rand() % points.size()];
while (true) {
// 找到当前中心与点集中所有点的最大距离平方
double max_distance_sq = 0.0;
for (const auto& point : points) {
if (distance_squared(point, center) > max_distance_sq) {
max_distance_sq = distance_squared(point, center);
}
}
// 如果最大距离平方小于等于当前中心距离自身的一半,说明已经找到了包围球
if (max_distance_sq <= distance_squared(center, center)) {
break;
} else {
// 更新中心为最大距离的点
center = {points[std::distance(points.begin(), std::min_element(points.begin(), points.end(),
[&](const Point& a, const Point& b) { return distance_squared(a, center) < distance_squared(b, center); })].x,
points[std::distance(points.begin(), std::min_element(points.begin(), points.end(),
[&](const Point& a, const Point& b) { return distance_squared(a, center) < distance_squared(b, center); })).y,
points[std::distance(points.begin(), std::min_element(points.begin(), points.end(),
[&](const Point& a, const Point& b) { return distance_squared(a, center) < distance_squared(b, center); })).z};
}
}
return center; // 返回最小包围球的中心
}
// 示例
int main() {
std::vector<Point> points = {{1, 1, 1}, {2, 2, 2}, {3, 3, 3}, ...}; // 假设你已经有了点集合
Point sphere_center = min_enclosing_sphere(points);
std::cout << "最小包围球的中心坐标: (" << sphere_center.x << ", " << sphere_center.y << ", " << sphere_center.z << ")" << std::endl;
return 0;
}
```
注意,这个实现并不保证找到的是真正的全局最小包围球,只是一个近似解。如果你需要保证结果的唯一性并寻找精确解,可能需要引入其他更复杂的优化技术。
阅读全文
相关推荐
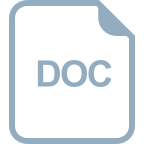
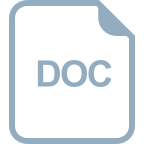
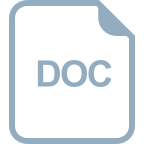
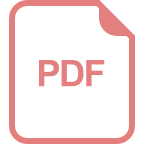
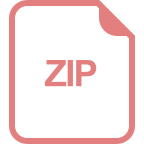
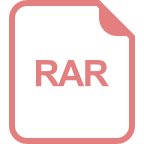
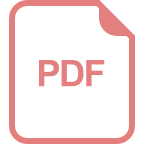
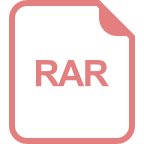
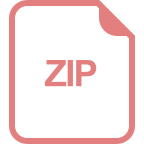
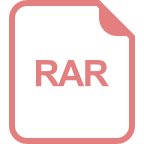
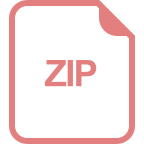
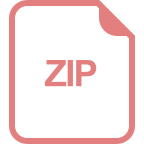
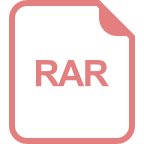
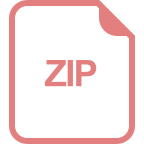
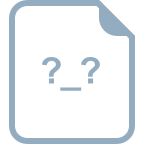
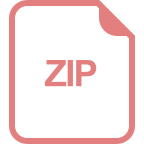
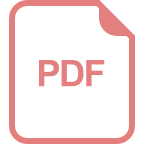